使用Vue实现包含id、英雄名字、类型、技能、血条及更改、删除、添加功能的英雄列表了。完整代码
时间: 2023-11-08 19:50:50 浏览: 49
以下是一个使用 Vue 实现英雄列表的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>英雄列表</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
}
th {
background-color: #4CAF50;
color: white;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
input[type=text], select {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
input[type=submit] {
background-color: #4CAF50;
color: white;
padding: 12px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
.container {
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
</style>
</head>
<body>
<div id="app" class="container">
<h2>英雄列表</h2>
<table>
<thead>
<tr>
<th>ID</th>
<th>英雄名字</th>
<th>类型</th>
<th>技能</th>
<th>血条</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(hero, index) in heroes" :key="index">
<td>{{ hero.id }}</td>
<td>{{ hero.name }}</td>
<td>{{ hero.type }}</td>
<td>{{ hero.skill }}</td>
<td><input type="range" min="0" max="100" v-model="hero.health"></td>
<td>
<button @click="editHero(index)">编辑</button>
<button @click="deleteHero(index)">删除</button>
</td>
</tr>
</tbody>
</table>
<br>
<h2 v-if="selectedHeroIndex !== null">编辑英雄</h2>
<form @submit.prevent="submitForm">
<label for="name">英雄名字</label>
<input type="text" id="name" v-model="selectedHero.name" required>
<label for="type">类型</label>
<select id="type" v-model="selectedHero.type">
<option value="战士">战士</option>
<option value="法师">法师</option>
<option value="刺客">刺客</option>
<option value="射手">射手</option>
<option value="辅助">辅助</option>
<option value="坦克">坦克</option>
</select>
<label for="skill">技能</label>
<input type="text" id="skill" v-model="selectedHero.skill" required>
<label for="health">血条</label>
<input type="range" id="health" min="0" max="100" v-model="selectedHero.health">
<input type="submit" value="提交">
</form>
<br>
<h2>添加英雄</h2>
<form @submit.prevent="addHero">
<label for="name">英雄名字</label>
<input type="text" id="name" v-model="newHero.name" required>
<label for="type">类型</label>
<select id="type" v-model="newHero.type">
<option value="战士">战士</option>
<option value="法师">法师</option>
<option value="刺客">刺客</option>
<option value="射手">射手</option>
<option value="辅助">辅助</option>
<option value="坦克">坦克</option>
</select>
<label for="skill">技能</label>
<input type="text" id="skill" v-model="newHero.skill" required>
<label for="health">血条</label>
<input type="range" id="health" min="0" max="100" v-model="newHero.health">
<input type="submit" value="添加">
</form>
</div>
<script>
new Vue({
el: '#app',
data: {
heroes: [
{
id: 1,
name: '盖伦',
type: '坦克',
skill: '勇气',
health: 100
},
{
id: 2,
name: '亚索',
type: '刺客',
skill: '疾风剑豪',
health: 100
},
{
id: 3,
name: '提莫',
type: '射手',
skill: '致盲吹箭',
health: 100
}
],
selectedHeroIndex: null,
selectedHero: null,
newHero: {
id: null,
name: '',
type: '',
skill: '',
health: 100
}
},
methods: {
editHero: function(index) {
this.selectedHeroIndex = index;
this.selectedHero = Object.assign({}, this.heroes[index]);
},
submitForm: function() {
if (this.selectedHeroIndex !== null) {
this.heroes.splice(this.selectedHeroIndex, 1, this.selectedHero);
this.selectedHeroIndex = null;
this.selectedHero = null;
}
},
deleteHero: function(index) {
this.heroes.splice(index, 1);
},
addHero: function() {
this.newHero.id = this.heroes.length + 1;
this.heroes.push(Object.assign({}, this.newHero));
this.newHero.name = '';
this.newHero.type = '';
this.newHero.skill = '';
this.newHero.health = 100;
}
}
});
</script>
</body>
</html>
```
该代码实现了以下功能:
- 显示英雄列表,每个英雄包含ID、名字、类型、技能和血条。
- 可以修改每个英雄的名字、类型、技能和血条,也可以删除英雄。
- 可以添加新英雄,新英雄的ID会自动递增。
相关推荐
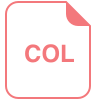
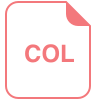
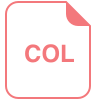
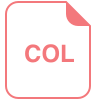
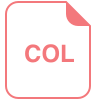









