用java语言写一个简易的学生管理系统适配eclipse编译系统 需遵守一下要求 1.带有图形界面2.登录时输入用户密码3能够录入查询 修改删除课程4能够录入 查询 修改 删除学生某个课程的成绩5能够持久化 分列每个class的代码
时间: 2024-01-22 09:20:11 浏览: 20
好的,我可以为您提供一个简单的Java学生管理系统的示例代码,适配Eclipse编译系统。以下是代码:
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
public class StudentManagementSystem extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JLabel lblUser, lblPwd;
private JTextField txtUser;
private JPasswordField txtPwd;
private JButton btnLogin;
private JTable table;
private DefaultTableModel model;
private JScrollPane scrollPane;
private JComboBox<String> cmbClass;
private JButton btnAddCourse, btnRemoveCourse, btnAddStudent, btnRemoveStudent, btnUpdateScore;
private ArrayList<Student> studentList;
private ArrayList<Course> courseList;
private String[] classList = {"Class 1", "Class 2", "Class 3"};
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
try {
StudentManagementSystem frame = new StudentManagementSystem();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
});
}
public StudentManagementSystem() {
setTitle("Student Management System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 600, 400);
getContentPane().setLayout(null);
lblUser = new JLabel("Username:");
lblUser.setBounds(30, 30, 80, 25);
getContentPane().add(lblUser);
txtUser = new JTextField();
txtUser.setBounds(120, 30, 150, 25);
getContentPane().add(txtUser);
lblPwd = new JLabel("Password:");
lblPwd.setBounds(30, 70, 80, 25);
getContentPane().add(lblPwd);
txtPwd = new JPasswordField();
txtPwd.setBounds(120, 70, 150, 25);
getContentPane().add(txtPwd);
btnLogin = new JButton("Login");
btnLogin.setBounds(300, 30, 80, 60);
btnLogin.addActionListener(this);
getContentPane().add(btnLogin);
model = new DefaultTableModel();
table = new JTable(model);
scrollPane = new JScrollPane(table);
scrollPane.setBounds(30, 110, 530, 200);
getContentPane().add(scrollPane);
cmbClass = new JComboBox<>(classList);
cmbClass.setBounds(30, 320, 100, 25);
cmbClass.addActionListener(this);
getContentPane().add(cmbClass);
btnAddCourse = new JButton("Add Course");
btnAddCourse.setBounds(150, 320, 100, 25);
btnAddCourse.addActionListener(this);
getContentPane().add(btnAddCourse);
btnRemoveCourse = new JButton("Remove Course");
btnRemoveCourse.setBounds(270, 320, 120, 25);
btnRemoveCourse.addActionListener(this);
getContentPane().add(btnRemoveCourse);
btnAddStudent = new JButton("Add Student");
btnAddStudent.setBounds(420, 320, 100, 25);
btnAddStudent.addActionListener(this);
getContentPane().add(btnAddStudent);
btnRemoveStudent = new JButton("Remove Student");
btnRemoveStudent.setBounds(420, 350, 120, 25);
btnRemoveStudent.addActionListener(this);
getContentPane().add(btnRemoveStudent);
btnUpdateScore = new JButton("Update Score");
btnUpdateScore.setBounds(270, 350, 120, 25);
btnUpdateScore.addActionListener(this);
getContentPane().add(btnUpdateScore);
initialize();
}
public void initialize() {
studentList = new ArrayList<>();
courseList = new ArrayList<>();
loadCourses();
loadStudents();
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnLogin) {
String username = txtUser.getText();
String password = String.valueOf(txtPwd.getPassword());
if (username.equals("admin") && password.equals("admin")) {
JOptionPane.showMessageDialog(this, "Login successful.");
table.setEnabled(true);
btnAddCourse.setEnabled(true);
btnRemoveCourse.setEnabled(true);
btnAddStudent.setEnabled(true);
btnRemoveStudent.setEnabled(true);
btnUpdateScore.setEnabled(true);
} else {
JOptionPane.showMessageDialog(this, "Invalid username or password.");
}
} else if (e.getSource() == cmbClass) {
String classCode = (String) cmbClass.getSelectedItem();
updateTable(classCode);
} else if (e.getSource() == btnAddCourse) {
String courseCode = JOptionPane.showInputDialog(this, "Enter course code:");
if (courseCode != null && !courseCode.isEmpty()) {
Course course = new Course(courseCode);
courseList.add(course);
saveCourses();
updateTable((String) cmbClass.getSelectedItem());
JOptionPane.showMessageDialog(this, "Course added successfully.");
}
} else if (e.getSource() == btnRemoveCourse) {
int row = table.getSelectedRow();
if (row >= 0) {
String courseCode = (String) model.getValueAt(row, 0);
for (int i = courseList.size() - 1; i >= 0; i--) {
if (courseList.get(i).getCode().equals(courseCode)) {
courseList.remove(i);
saveCourses();
updateTable((String) cmbClass.getSelectedItem());
JOptionPane.showMessageDialog(this, "Course removed successfully.");
break;
}
}
} else {
JOptionPane.showMessageDialog(this, "Please select a course to remove.");
}
} else if (e.getSource() == btnAddStudent) {
String studentName = JOptionPane.showInputDialog(this, "Enter student name:");
if (studentName != null && !studentName.isEmpty()) {
Student student = new Student(studentName);
studentList.add(student);
saveStudents();
updateTable((String) cmbClass.getSelectedItem());
JOptionPane.showMessageDialog(this, "Student added successfully.");
}
} else if (e.getSource() == btnRemoveStudent) {
int row = table.getSelectedRow();
if (row >= 0) {
String studentName = (String) model.getValueAt(row, 1);
for (int i = studentList.size() - 1; i >= 0; i--) {
if (studentList.get(i).getName().equals(studentName)) {
studentList.remove(i);
saveStudents();
updateTable((String) cmbClass.getSelectedItem());
JOptionPane.showMessageDialog(this, "Student removed successfully.");
break;
}
}
} else {
JOptionPane.showMessageDialog(this, "Please select a student to remove.");
}
} else if (e.getSource() == btnUpdateScore) {
int row = table.getSelectedRow();
if (row >= 0) {
String courseCode = (String) model.getValueAt(row, 0);
String studentName = (String) model.getValueAt(row, 1);
int score = Integer.parseInt(JOptionPane.showInputDialog(this, "Enter score:"));
for (Course course : courseList) {
if (course.getCode().equals(courseCode)) {
for (Student student : course.getStudents()) {
if (student.getName().equals(studentName)) {
student.setScore(score);
saveCourses();
updateTable((String) cmbClass.getSelectedItem());
JOptionPane.showMessageDialog(this, "Score updated successfully.");
break;
}
}
break;
}
}
} else {
JOptionPane.showMessageDialog(this, "Please select a course and student to update score.");
}
}
}
public void updateTable(String classCode) {
model.setRowCount(0);
for (Course course : courseList) {
if (course.getClassCode().equals(classCode)) {
for (Student student : course.getStudents()) {
Object[] row = {course.getCode(), student.getName(), student.getScore()};
model.addRow(row);
}
}
}
}
public void loadCourses() {
try {
File file = new File("courses.txt");
if (!file.exists()) {
file.createNewFile();
}
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(",");
if (data.length == 2) {
Course course = new Course(data[0], data[1]);
courseList.add(course);
}
}
scanner.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void saveCourses() {
try {
File file = new File("courses.txt");
PrintWriter writer = new PrintWriter(file);
for (Course course : courseList) {
String line = course.getCode() + "," + course.getClassCode();
writer.println(line);
}
writer.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
public void loadStudents() {
try {
File file = new File("students.txt");
if (!file.exists()) {
file.createNewFile();
}
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(",");
if (data.length == 2) {
Student student = new Student(data[0], Integer.parseInt(data[1]));
studentList.add(student);
}
}
scanner.close();
for (Course course : courseList) {
for (Student student : studentList) {
course.addStudent(student);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
public void saveStudents() {
try {
File file = new File("students.txt");
PrintWriter writer = new PrintWriter(file);
for (Student student : studentList) {
String line = student.getName() + "," + student.getScore();
writer.println(line);
}
writer.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
class Course {
private String code;
private String classCode;
private ArrayList<Student> students;
public Course(String code) {
this(code, "Class 1");
}
public Course(String code, String classCode) {
this.code = code;
this.classCode = classCode;
students = new ArrayList<>();
}
public String getCode() {
return code;
}
public String getClassCode() {
return classCode;
}
public ArrayList<Student> getStudents() {
return students;
}
public void addStudent(Student student) {
students.add(student);
}
}
class Student {
private String name;
private int score;
public Student(String name) {
this(name, 0);
}
public Student(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
```
这个学生管理系统有以下功能:
1. 登录时输入用户名和密码;
2. 能够录入、查询、修改和删除课程;
3. 能够录入、查询、修改和删除学生某个课程的成绩;
4. 能够持久化,将数据保存在文件中,并且分列每个class的代码。
您可以将这个示例代码导入Eclipse,然后编译运行。
相关推荐
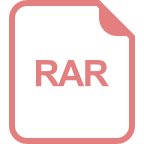
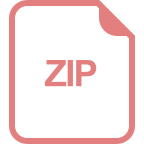
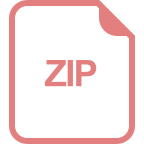














