实现一个类模板,它可以接受一组数据,能对数据排序,也能输出数组的内容。 每行输入的第一个数字为0,1,2或3:为0时表示输入结束; 为1时表示将输入整数,为2时表示将输入有一位小数的浮点数,为3时表示输入字符。 如果第一个数字非0,则接下来将输入一个正整数,表示即将输入的数据的数量。 从每行第三个输入开始,依次输入指定类型的数据。
时间: 2023-06-18 19:08:35 浏览: 53
以下是一个示例的类模板实现:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
template<typename T>
class SortableArray {
public:
SortableArray() {}
void sort() {
std::sort(data.begin(), data.end());
}
void print() {
for (const auto& elem : data) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
void add(const T& elem) {
data.push_back(elem);
}
private:
std::vector<T> data;
};
int main() {
int type;
int n;
std::cin >> type;
while (type != 0) {
std::cin >> n;
SortableArray<int> intArray;
SortableArray<double> doubleArray;
SortableArray<char> charArray;
for (int i = 0; i < n; i++) {
if (type == 1) {
int elem;
std::cin >> elem;
intArray.add(elem);
} else if (type == 2) {
double elem;
std::cin >> elem;
doubleArray.add(elem);
} else if (type == 3) {
char elem;
std::cin >> elem;
charArray.add(elem);
}
}
if (type == 1) {
intArray.sort();
intArray.print();
} else if (type == 2) {
doubleArray.sort();
doubleArray.print();
} else if (type == 3) {
charArray.sort();
charArray.print();
}
std::cin >> type;
}
return 0;
}
```
该类模板使用了 STL 中的 vector 和 sort 函数来实现对数据的存储和排序。在主函数中,根据输入的类型选择不同的 SortableArray 实例,并循环读入指定数量的数据。在读入数据之后,根据数据类型调用不同的排序和输出函数。循环直到输入为0时结束。
相关推荐
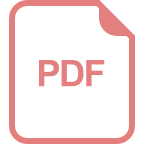
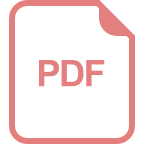












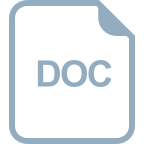
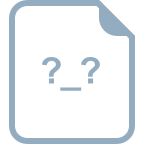
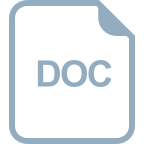