使用C语言编写一个程序,输入两个英文句子,每个句子里英文单词之间用空格分隔,输出它们最长的公共单词。
时间: 2024-04-10 13:27:20 浏览: 23
您好!您可以使用C语言编写一个程序来实现这个功能。以下是一个示例程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LENGTH 100
void findCommonWord(char* sentence1, char* sentence2, char* result) {
char* word1 = strtok(sentence1, " ");
char* longestCommonWord = "";
while (word1 != NULL) {
char* word2 = strtok(sentence2, " ");
while (word2 != NULL) {
if (strcmp(word1, word2) == 0 && strlen(word1) > strlen(longestCommonWord)) {
longestCommonWord = word1;
}
word2 = strtok(NULL, " ");
}
word1 = strtok(NULL, " ");
}
strcpy(result, longestCommonWord);
}
int main() {
char sentence1[MAX_LENGTH];
char sentence2[MAX_LENGTH];
char commonWord[MAX_LENGTH];
printf("Enter the first sentence: ");
fgets(sentence1, sizeof(sentence1), stdin);
sentence1[strcspn(sentence1, "\n")] = '\0';
printf("Enter the second sentence: ");
fgets(sentence2, sizeof(sentence2), stdin);
sentence2[strcspn(sentence2, "\n")] = '\0';
findCommonWord(sentence1, sentence2, commonWord);
if (strlen(commonWord) > 0) {
printf("The longest common word is: %s\n", commonWord);
} else {
printf("No common word found.\n");
}
return 0;
}
```
这个程序使用了`strtok`函数来将句子拆分成单词,并使用`strcmp`函数来比较两个单词是否相等。最长的公共单词是通过比较单词长度来确定的。
相关推荐
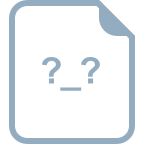














