编写程序,输入n个学生的学号和成绩(有1位小数),按成绩从高到低排序输出,n小于50,学号小于10位。
时间: 2024-02-12 07:02:27 浏览: 16
好的,这是一个简单的C语言程序,可以实现你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 50
#define MAX_ID_LEN 10
// 学生结构体
typedef struct {
char id[MAX_ID_LEN+1]; // 学号
double score; // 成绩
} Student;
// 从高到低排序函数
int cmp(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
if (s1->score < s2->score) {
return 1;
} else if (s1->score > s2->score) {
return -1;
} else {
return strcmp(s1->id, s2->id); // 成绩相等按学号从小到大排序
}
}
int main() {
int n, i;
Student students[MAX_N];
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%s %lf", students[i].id, &students[i].score);
}
// 使用qsort函数从高到低排序
qsort(students, n, sizeof(Student), cmp);
// 输出排序后的结果
for (i = 0; i < n; i++) {
printf("%s %.1lf\n", students[i].id, students[i].score);
}
return 0;
}
```
这个程序首先定义了一个学生结构体,包含学号和成绩两个字段。在输入时,使用循环遍历n个学生,依次读入学号和成绩。在排序时,使用qsort函数从高到低排序,如果成绩相等则按照学号从小到大排序。在输出时,使用循环遍历n个学生,按照顺序输出学号和成绩。
相关推荐
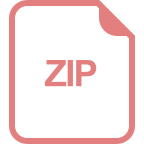
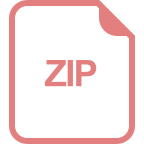














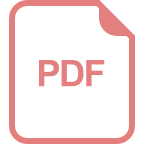