vector.erase()之后的size()
时间: 2023-11-11 18:06:48 浏览: 126
在使用 `vector.erase()` 删除元素后,`vector` 的 `size()` 会减小,因为元素已经被删除了。具体来说,如果删除单个元素,则 `size()` 会减 1;如果删除多个元素,则 `size()` 会减去删除元素的个数。
例如,如果有一个 `vector<int> v`,初始时包含 5 个元素,且要删除第 2 个元素,则可以使用以下代码:
```c++
vector<int>::iterator it = v.begin() + 1; // 获取第 2 个元素的迭代器
v.erase(it); // 删除第 2 个元素
cout << v.size() << endl; // 输出 4
```
在上述代码中,`v.size()` 在删除元素后为 4。
需要注意的是,如果在删除元素时使用了迭代器,则删除元素后迭代器会失效,因此需要重新获取迭代器。如果在删除元素后继续使用失效的迭代器,则会导致不可预期的行为。
相关问题
clusters.erase
非常抱歉,我的回答里有误,`clusters.erase`应该改为`clusters.erase(clusters.begin() + minCluster2)`。这是因为 `clusters` 是一个 `vector`,我们需要使用 `vector` 的 `erase` 函数来删除其中的元素。
完整的代码如下:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <limits>
using namespace std;
struct DataPoint {
double value;
int cluster;
};
double getDistance(const DataPoint& p1, const DataPoint& p2) {
return fabs(p1.value - p2.value);
}
void mergeClusters(vector<DataPoint>& data, int cluster1, int cluster2) {
for (auto& point : data) {
if (point.cluster == cluster2) {
point.cluster = cluster1;
}
}
}
void hierarchicalClustering(vector<DataPoint>& data) {
int n = data.size();
vector<int> clusters(n);
for (int i = 0; i < n; ++i) {
clusters[i] = i;
}
while (n > 1) {
double minDist = numeric_limits<double>::max();
int minCluster1 = -1, minCluster2 = -1;
for (int i = 0; i < n-1; ++i) {
for (int j = i+1; j < n; ++j) {
double dist = getDistance(data[i], data[j]);
if (dist < minDist) {
minDist = dist;
minCluster1 = clusters[i];
minCluster2 = clusters[j];
}
}
}
mergeClusters(data, minCluster1, minCluster2);
clusters.erase(clusters.begin() + minCluster2);
for (auto& point : data) {
if (point.cluster > minCluster2) {
--point.cluster;
}
}
--n;
}
}
int main() {
vector<DataPoint> data = {{1.0, 0}, {2.0, 1}, {5.0, 2}, {6.0, 3}, {7.0, 4}, {8.0, 5}};
hierarchicalClustering(data);
for (const auto& point : data) {
cout << point.value << " " << point.cluster << endl;
}
return 0;
}
```
该程序可以将输入的数据按照差值进行聚类,并输出每个数据点所属的簇的编号。输入的数据是一个包含六个数据点的向量,每个数据点包含数据本身和所属的簇的编号。在程序中,我们首先对每个数据点初始化一个簇的编号,然后进行层次聚类,最终得到每个数据点所属的最终簇的编号。
vector.xy()
根据提供的引用内容,vector是C++标准模板库(STL)中的一个容器,用于存储一组元素。下面是关于vector的基本使用方法:
1. 初始化vector:
```cpp
vector<int> v; // 初始化一个空的vector
vector<int> v(10, 1); // 初始化一个包含10个值为1的元素的vector
vector<int> v(v1); // 将另一个vector v1 的元素拷贝给v
vector<int> v(v1.begin(), v2.end()); // 将v1中从第一个元素到最后一个元素(不包括最后一个元素)的范围拷贝给v
```
2. 插入元素到vector中:
```cpp
v.push_back(5); // 在vector的末尾插入一个元素5
v.insert(v.begin() + 2, 10); // 在vector的第3个位置(索引为2)插入一个元素10
```
3. 遍历vector中的元素:
```cpp
for (int i = 0; i < v.size(); i++) {
cout << v[i] << " "; // 输出vector中的每个元素
}
```
4. 删除vector中的元素:
```cpp
v.pop_back(); // 删除vector的最后一个元素
v.erase(v.begin() + 2); // 删除vector的第3个元素(索引为2)
```
相关推荐
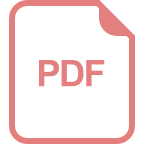
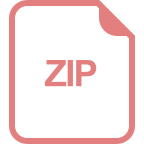
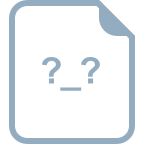













