用矩形框选中图像的一个区域,这个区域有两条相邻的边,怎么得到这两条边的夹角呢,用halcon C++实现
时间: 2023-12-14 21:37:41 浏览: 95
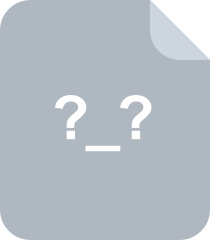
三角形的两边及其夹角求面积.cpp
可以使用HoughLines函数检测出图像中的直线,并计算相邻两条线段的夹角来获取这两条边的夹角。具体步骤如下:
1. 使用gen_rectangle2函数生成矩形区域的ROI。
2. 使用HoughLines函数检测图像中的直线,并将结果存储在Lines中。
```
HoughLines(RectangleImage, 3, HTuple(0), HTuple(0.0175), HTuple(30), HTuple(10), HTuple("positive"), &Lines);
```
3. 遍历Lines,找到矩形区域内的直线,并计算相邻两条线段的夹角。
```
HTuple rowBegin, colBegin, rowEnd, colEnd;
HTuple angle, deltaAngle;
for (int i = 0; i < Lines.Length(); i++)
{
TupleGet(Lines[i], 0, &rowBegin);
TupleGet(Lines[i], 1, &colBegin);
TupleGet(Lines[i], 2, &rowEnd);
TupleGet(Lines[i], 3, &colEnd);
if (TupleLength(rowBegin) == 1 && TupleLength(colBegin) == 1 && TupleLength(rowEnd) == 1 && TupleLength(colEnd) == 1)
{
if (rowBegin > row1 && rowBegin < row2 && rowEnd > row1 && rowEnd < row2 && colBegin > col1 && colBegin < col2 && colEnd > col1 && colEnd < col2)
{
TupleAngle(HTuple(rowBegin, colBegin, rowEnd, colEnd), &angle);
deltaAngle = angle - preAngle;
if (deltaAngle < 0)
{
deltaAngle += HTuple(360);
}
if (deltaAngle > 180)
{
deltaAngle = HTuple(360) - deltaAngle;
}
if (deltaAngle > maxAngle)
{
maxAngle = deltaAngle;
}
preAngle = angle;
}
}
}
```
其中,row1、col1、row2、col2分别是矩形区域的左上和右下坐标,preAngle是上一条线段的角度,maxAngle是相邻两条线段的最大夹角。
4. 最终得到的maxAngle即为这两条边的夹角。
完整代码如下:
```
// 生成矩形区域的ROI
gen_rectangle2(&RectangleROI, HTuple(200), HTuple(200), HTuple(300), HTuple(300), HTuple(0));
// 将ROI放入图像中
reduce_domain(RectangleImage, RectangleROI, &RectangleImageReduced);
// 检测直线并存储结果
HoughLines(RectangleImageReduced, 3, HTuple(0), HTuple(0.0175), HTuple(30), HTuple(10), HTuple("positive"), &Lines);
// 遍历直线,计算夹角
HTuple rowBegin, colBegin, rowEnd, colEnd;
HTuple angle, deltaAngle;
HTuple row1 = 200, col1 = 200, row2 = 300, col2 = 300;
HTuple preAngle = 0, maxAngle = 0;
for (int i = 0; i < Lines.Length(); i++)
{
TupleGet(Lines[i], 0, &rowBegin);
TupleGet(Lines[i], 1, &colBegin);
TupleGet(Lines[i], 2, &rowEnd);
TupleGet(Lines[i], 3, &colEnd);
if (TupleLength(rowBegin) == 1 && TupleLength(colBegin) == 1 && TupleLength(rowEnd) == 1 && TupleLength(colEnd) == 1)
{
if (rowBegin > row1 && rowBegin < row2 && rowEnd > row1 && rowEnd < row2 && colBegin > col1 && colBegin < col2 && colEnd > col1 && colEnd < col2)
{
TupleAngle(HTuple(rowBegin, colBegin, rowEnd, colEnd), &angle);
deltaAngle = angle - preAngle;
if (deltaAngle < 0)
{
deltaAngle += HTuple(360);
}
if (deltaAngle > 180)
{
deltaAngle = HTuple(360) - deltaAngle;
}
if (deltaAngle > maxAngle)
{
maxAngle = deltaAngle;
}
preAngle = angle;
}
}
}
// 显示结果
disp_image(RectangleImage, WindowHandle);
disp_message(WindowHandle, HTuple(50), HTuple(50), HTuple("Angle: ") + maxAngle, HTuple("image"), HTuple("black"), HTuple(), HTuple());
```
阅读全文
相关推荐
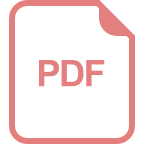
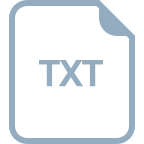
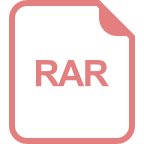
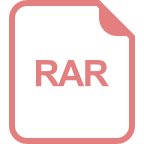
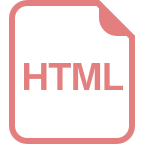
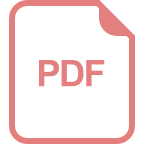
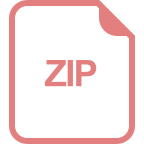
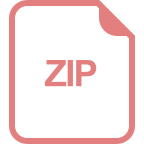
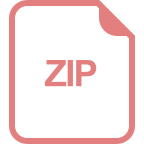
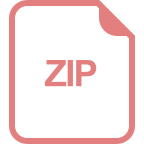
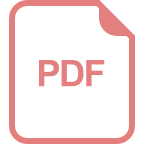
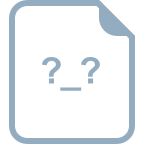
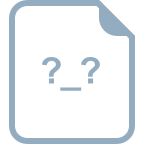
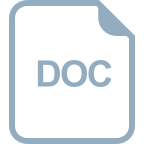