在Androidstudio中设计一个简单学生选课系统并给出代码以及XML代码,在这个应用程序中有两个Activity界面,分别是对课程进行操作时的CourseActivity界面,以及对学生操作的PersonActivity界面。在CourseActivity界面可以对课程进行添加,删除以及跳转到PersonActivity功能和通过ListView将所有课程显示在页面上,在PersonActivity中可以对person表数据进行增、删、改等操作和通过ListView将所有学生信息显示在页面上,在这个工程中有两个实体类(Person.java、Course.java)和一个扩展类(Relation.java),Person.java类定义了学生的属性信息,Course.java类定义了课程的属性对象,而Relation.java类是学生和课程之间的关系类实现了学生和课程之间的多对多存储关系。三个类的属性定义如下,(1)person类的定义为:id(学生号),myname(学生名),myclass(学生班级),(2)Course类:id(课程号)、name(课程名)、obj(上课对象)、phone(班长电话),(3)Relation类:studentId(学生id)、courseId(课程Id)。定义三个数据表,表结构分别为:student表、course表、relations表,通过定义DBOpenHelper类继承SQLiteOpenHelper类来创建数据库,使用SQLite存储数据
时间: 2024-02-01 12:13:39 浏览: 20
这是一个简单的学生选课系统的代码和XML代码,其中包含两个Activity界面,一个CourseActivity界面和一个PersonActivity界面。
1. CourseActivity.java
```
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import java.util.ArrayList;
public class CourseActivity extends AppCompatActivity {
private ListView listView;
private ArrayList<Course> courseList = new ArrayList<>();
private ArrayAdapter<Course> adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_course);
listView = (ListView) findViewById(R.id.listView);
adapter = new ArrayAdapter<Course>(this, android.R.layout.simple_list_item_1, courseList);
listView.setAdapter(adapter);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(CourseActivity.this, PersonActivity.class);
intent.putExtra("courseId", courseList.get(position).getId());
startActivity(intent);
}
});
}
public void addCourse(View view) {
// TODO: 添加课程
}
public void deleteCourse(View view) {
// TODO: 删除课程
}
}
```
2. activity_course.xml
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".CourseActivity">
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/button_layout" />
<LinearLayout
android:id="@+id/button_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="horizontal">
<Button
android:id="@+id/add_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="addCourse"
android:text="添加" />
<Button
android:id="@+id/delete_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="deleteCourse"
android:text="删除" />
</LinearLayout>
</RelativeLayout>
```
3. PersonActivity.java
```
import android.content.ContentValues;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Toast;
import java.util.ArrayList;
public class PersonActivity extends AppCompatActivity {
private ListView listView;
private ArrayList<Person> personList = new ArrayList<>();
private ArrayAdapter<Person> adapter;
private int courseId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_person);
listView = (ListView) findViewById(R.id.listView);
adapter = new ArrayAdapter<Person>(this, android.R.layout.simple_list_item_1, personList);
listView.setAdapter(adapter);
courseId = getIntent().getIntExtra("courseId", 0);
loadPersonList();
}
public void addPerson(View view) {
EditText idEditText = (EditText) findViewById(R.id.id_editText);
EditText nameEditText = (EditText) findViewById(R.id.name_editText);
EditText classEditText = (EditText) findViewById(R.id.class_editText);
String id = idEditText.getText().toString();
String name = nameEditText.getText().toString();
String myclass = classEditText.getText().toString();
if (id.isEmpty() || name.isEmpty() || myclass.isEmpty()) {
Toast.makeText(this, "请填写完整信息", Toast.LENGTH_SHORT).show();
return;
}
SQLiteDatabase db = new DBOpenHelper(this).getWritableDatabase();
ContentValues values = new ContentValues();
values.put("id", id);
values.put("myname", name);
values.put("myclass", myclass);
db.insert("person", null, values);
values.clear();
values.put("studentId", id);
values.put("courseId", courseId);
db.insert("relations", null, values);
loadPersonList();
idEditText.setText("");
nameEditText.setText("");
classEditText.setText("");
}
public void deletePerson(View view) {
// TODO: 删除学生
}
public void updatePerson(View view) {
// TODO: 更新学生信息
}
private void loadPersonList() {
personList.clear();
SQLiteDatabase db = new DBOpenHelper(this).getReadableDatabase();
Cursor cursor = db.rawQuery("SELECT * FROM person, relations WHERE person.id = relations.studentId AND relations.courseId = " + courseId, null);
while (cursor.moveToNext()) {
Person person = new Person();
person.setId(cursor.getString(cursor.getColumnIndex("id")));
person.setName(cursor.getString(cursor.getColumnIndex("myname")));
person.setMyclass(cursor.getString(cursor.getColumnIndex("myclass")));
personList.add(person);
}
adapter.notifyDataSetChanged();
}
}
```
4. activity_person.xml
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<EditText
android:id="@+id/id_editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="学号" />
<EditText
android:id="@+id/name_editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="姓名" />
<EditText
android:id="@+id/class_editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="班级" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/add_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="addPerson"
android:text="添加" />
<Button
android:id="@+id/delete_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="deletePerson"
android:text="删除" />
<Button
android:id="@+id/update_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="updatePerson"
android:text="更新" />
</LinearLayout>
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
</LinearLayout>
```
5. DBOpenHelper.java
```
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DBOpenHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "course.db";
private static final int DATABASE_VERSION = 1;
public DBOpenHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE IF NOT EXISTS course(" +
"id INTEGER PRIMARY KEY," +
"name TEXT," +
"obj TEXT," +
"phone TEXT)");
db.execSQL("CREATE TABLE IF NOT EXISTS person(" +
"id TEXT PRIMARY KEY," +
"myname TEXT," +
"myclass TEXT)");
db.execSQL("CREATE TABLE IF NOT EXISTS relations(" +
"id INTEGER PRIMARY KEY," +
"studentId TEXT," +
"courseId INTEGER)");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS course");
db.execSQL("DROP TABLE IF EXISTS person");
db.execSQL("DROP TABLE IF EXISTS relations");
onCreate(db);
}
}
```
相关推荐
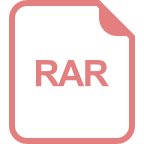
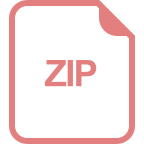















