vue上传图片的过程
时间: 2023-07-28 07:19:47 浏览: 55
好的,下面是Vue上传图片的大致过程:
1. 安装并引入第三方组件库,如vue-axios、element-ui等。其中vue-axios用于发送http请求,element-ui的el-upload组件用于上传图片。
```javascript
npm install --save vue-axios element-ui
```
```javascript
import axios from 'axios'
import VueAxios from 'vue-axios'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
Vue.use(VueAxios, axios)
Vue.use(ElementUI)
```
2. 在模板中使用el-upload组件,并设置相应属性,如上传地址、文件类型、文件大小等。
```html
<template>
<el-upload
action="/upload"
:before-upload="beforeUpload"
:on-success="onSuccess"
:on-error="onError"
:file-list="fileList"
:auto-upload="false"
list-type="picture-card"
:limit="3"
:max-size="2048"
:accept="'image/*'"
>
<i class="el-icon-plus"></i>
<div class="el-upload__text">Upload</div>
</el-upload>
</template>
```
其中,action属性指定上传地址;before-upload方法用于上传前的处理;on-success方法用于上传成功后的处理;on-error方法用于上传失败后的处理;file-list属性用于设置已上传文件列表;auto-upload属性用于是否自动上传;list-type属性用于设置展示方式;limit属性用于设置最多上传文件数量;max-size属性用于设置最大文件大小限制;accept属性用于设置可上传的文件类型。
3. 编写beforeUpload方法,在该方法中进行文件大小、文件类型等的校验。
```javascript
beforeUpload(file) {
const isJPG = file.type === 'image/jpeg'
const isPNG = file.type === 'image/png'
const isGIF = file.type === 'image/gif'
const isLt2M = file.size / 1024 / 1024 < 2
if (!isJPG && !isPNG && !isGIF) {
this.$message.error('上传图片只能是 JPG/PNG/GIF 格式!')
return false
}
if (!isLt2M) {
this.$message.error('上传图片大小不能超过 2MB!')
return false
}
return true
}
```
4. 编写onSuccess方法,用于接收上传成功后的响应数据。
```javascript
onSuccess(response, file, fileList) {
this.$message.success('上传成功!')
this.fileList = fileList
}
```
5. 编写onError方法,用于接收上传失败后的响应数据。
```javascript
onError(err, file, fileList) {
this.$message.error('上传失败!')
}
```
6. 最后,编写上传方法,将文件上传至后台服务器。
```javascript
upload() {
const formData = new FormData()
this.fileList.forEach(file => {
formData.append('file', file.raw)
})
this.axios.post('/upload', formData).then(response => {
console.log(response.data)
})
}
```
其中,将文件添加到formData中,并使用axios发送post请求到/upload接口,后台服务器可以通过解析multipart/form-data类型的请求来获取上传的文件内容。
以上就是Vue上传图片的大致过程,希望对您有所帮助。
相关推荐
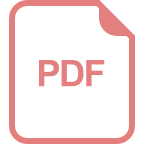














