Apache 的 FTPClient如何上传图片到不同的文件夹?需判断文件夹是否存在
时间: 2023-12-13 21:02:58 浏览: 61
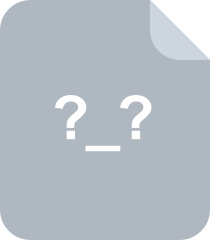
FTPClient 文件上传
使用 Apache 的 FTPClient 上传图片到不同的文件夹,可以按照以下步骤进行:
1. 首先连接到 FTP 服务器,使用 FTPClient 的 `connect()` 和 `login()` 方法。
```java
FTPClient ftpClient = new FTPClient();
ftpClient.connect(server, port);
ftpClient.login(username, password);
```
2. 判断需要上传的文件夹是否存在,可以使用 FTPClient 的 `changeWorkingDirectory()` 方法进入到该文件夹,如果返回 `false` 则说明该文件夹不存在,需要创建该文件夹。
```java
String remoteDirPath = "/path/to/remote/directory";
boolean success = ftpClient.changeWorkingDirectory(remoteDirPath);
if (!success) {
ftpClient.makeDirectory(remoteDirPath);
ftpClient.changeWorkingDirectory(remoteDirPath);
}
```
3. 上传图片文件,使用 FTPClient 的 `storeFile()` 方法上传文件。
```java
String localFilePath = "/path/to/local/file/image.jpg";
String remoteFileName = "image.jpg";
InputStream inputStream = new FileInputStream(localFilePath);
boolean success = ftpClient.storeFile(remoteFileName, inputStream);
inputStream.close();
```
4. 最后关闭连接,使用 FTPClient 的 `logout()` 和 `disconnect()` 方法。
```java
ftpClient.logout();
ftpClient.disconnect();
```
完整的代码示例:
```java
FTPClient ftpClient = new FTPClient();
ftpClient.connect(server, port);
ftpClient.login(username, password);
String remoteDirPath = "/path/to/remote/directory";
boolean success = ftpClient.changeWorkingDirectory(remoteDirPath);
if (!success) {
ftpClient.makeDirectory(remoteDirPath);
ftpClient.changeWorkingDirectory(remoteDirPath);
}
String localFilePath = "/path/to/local/file/image.jpg";
String remoteFileName = "image.jpg";
InputStream inputStream = new FileInputStream(localFilePath);
success = ftpClient.storeFile(remoteFileName, inputStream);
inputStream.close();
ftpClient.logout();
ftpClient.disconnect();
```
注意:在实际开发中,需要对异常进行处理并关闭资源。
阅读全文
相关推荐

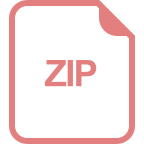
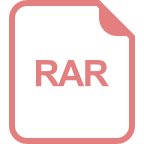
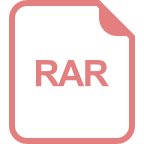
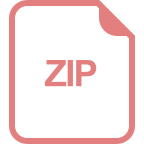
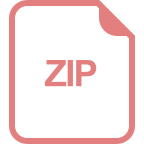
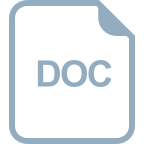



