import javax.print.*; import javax.print.attribute.*; import javax.print.attribute.standard.*; public class HPPrinter { public static void main(String[] args) { // 获取系统中所有打印机的名称 PrintService[] services = PrintServiceLookup.lookupPrintServices(null, null); for (PrintService service : services) { System.out.println(service.getName()); } // 选择要连接的打印机 String printerName = "Your Printer Name"; PrintService printerService = null; for (PrintService service : services) { if (service.getName().equals(printerName)) { printerService = service; break; } } if (printerService != null) { try { // 创建打印作业 DocPrintJob printJob = printerService.createPrintJob(); // 设置打印参数 PrintRequestAttributeSet attributes = new HashPrintRequestAttributeSet(); attributes.add(new Copies(1)); // 设置打印模式为彩色 String colorMode = "ON"; String command = "\u001B%-12345X@PJL SET COLOR=" + colorMode + "\r\n"; byte[] bytes = command.getBytes("ISO-8859-1"); DocFlavor flavor = DocFlavor.BYTE_ARRAY.AUTOSENSE; Doc doc = new SimpleDoc(bytes, flavor, null); // 提交打印作业 printJob.print(doc, attributes); } catch (Exception e) { e.printStackTrace(); } } else { System.out.println("Printer not found."); } } } 这段代码添加获取文件并使用打印机可以打印
时间: 2024-02-11 07:16:01 浏览: 115
好的,可以在上面的代码中添加获取文件并使用打印机的功能。可以参考下面的代码:
```java
import javax.print.*;
import javax.print.attribute.*;
import javax.print.attribute.standard.*;
import java.io.*;
public class HPPrinter {
public static void main(String[] args) {
// 获取系统中所有打印机的名称
PrintService[] services = PrintServiceLookup.lookupPrintServices(null, null);
for (PrintService service : services) {
System.out.println(service.getName());
}
// 选择要连接的打印机
String printerName = "Your Printer Name";
PrintService printerService = null;
for (PrintService service : services) {
if (service.getName().equals(printerName)) {
printerService = service;
break;
}
}
if (printerService != null) {
try {
// 获取要打印的文件
String filePath = "C:/test.pdf";
FileInputStream fileInputStream = new FileInputStream(filePath);
// 创建打印作业
DocPrintJob printJob = printerService.createPrintJob();
// 设置打印参数
PrintRequestAttributeSet attributes = new HashPrintRequestAttributeSet();
attributes.add(new Copies(1));
// 设置打印模式为彩色
String colorMode = "ON";
String command = "\u001B%-12345X@PJL SET COLOR=" + colorMode + "\r\n";
byte[] bytes = command.getBytes("ISO-8859-1");
DocFlavor flavor = DocFlavor.BYTE_ARRAY.AUTOSENSE;
Doc doc = new SimpleDoc(bytes, flavor, null);
// 提交打印作业
Doc docToPrint = new SimpleDoc(fileInputStream, DocFlavor.INPUT_STREAM.AUTOSENSE, null);
printJob.print(doc, attributes);
printJob.print(docToPrint, attributes);
fileInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
} else {
System.out.println("Printer not found.");
}
}
}
```
其中,`FileInputStream`用来读取要打印的文件,`Doc`用来发送打印机命令,`DocFlavor.INPUT_STREAM.AUTOSENSE`用来告诉打印机要打印的是一个输入流。最后,将要打印的文件和发送打印机命令的`Doc`一起提交给打印作业即可。
阅读全文
相关推荐
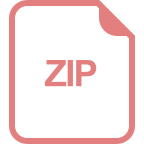
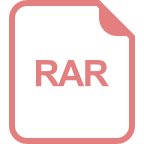
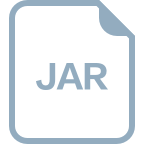
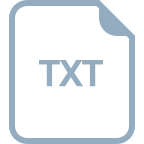
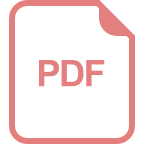
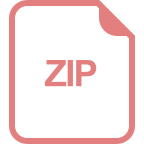
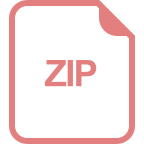
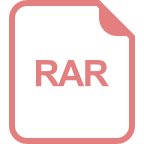
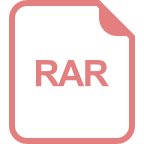
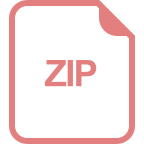
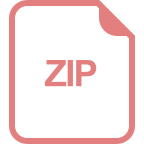
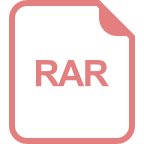
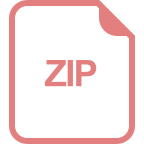
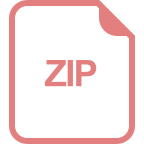
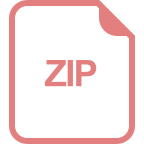
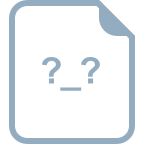