课程目标4:能的够从23种软件设计模式中选择恰当的软件设计模式对复杂的软件开发项目进行分析、设计、开发、测试和验证 题目:设计一个简单的咖啡店系统,需要支特下单、制作咖啡、送餐等功能。其中,咖啡分为浓缩咖啡、美式咖啡和拿铁咖啡, 每种咖啡可添不同口 味的糖浆、牛奶和奶泡。咖啡的成本根据咖啡类型和添加的配料不同而有所区别。请使用面向对象的思想,并使用适当的设计模式来实现该咖啡店系 统。请提供类的设计和相关的代码实现。(要求先说明采用了那些模式,再设计代码,把代码复制发到答题框。)
时间: 2024-03-08 20:45:45 浏览: 34
本题可以采用以下设计模式:
1. 工厂模式:根据用户选择的咖啡类型和配料,生产相应的咖啡对象。
2. 装饰器模式:用于动态扩展咖啡对象的行为,比如添加糖浆、牛奶和奶泡等。
3. 策略模式:用于根据用户的选择计算咖啡的成本。
以下是代码实现:
```python
# 咖啡类
class Coffee:
def get_cost(self):
pass
def get_description(self):
pass
# 浓缩咖啡类
class Espresso(Coffee):
def __init__(self):
self._description = 'Espresso'
def get_cost(self):
return 1.99
def get_description(self):
return self._description
# 美式咖啡类
class Americano(Coffee):
def __init__(self):
self._description = 'Americano'
def get_cost(self):
return 0.99
def get_description(self):
return self._description
# 拿铁咖啡类
class Latte(Coffee):
def __init__(self):
self._description = 'Latte'
def get_cost(self):
return 2.49
def get_description(self):
return self._description
# 装饰器类
class Decorator(Coffee):
def __init__(self, coffee):
self._coffee = coffee
def get_cost(self):
return self._coffee.get_cost()
def get_description(self):
return self._coffee.get_description()
# 糖浆装饰器类
class SyrupDecorator(Decorator):
def __init__(self, coffee, syrup):
super().__init__(coffee)
self._syrup = syrup
def get_cost(self):
return super().get_cost() + 0.5
def get_description(self):
return super().get_description() + f', {self._syrup} syrup'
# 牛奶装饰器类
class MilkDecorator(Decorator):
def __init__(self, coffee, milk):
super().__init__(coffee)
self._milk = milk
def get_cost(self):
return super().get_cost() + 0.7
def get_description(self):
return super().get_description() + f', {self._milk} milk'
# 奶泡装饰器类
class FoamDecorator(Decorator):
def __init__(self, coffee):
super().__init__(coffee)
def get_cost(self):
return super().get_cost() + 0.3
def get_description(self):
return super().get_description() + ', foam'
# 咖啡工厂类
class CoffeeFactory:
@staticmethod
def create_coffee(coffee_type):
if coffee_type == 'espresso':
return Espresso()
elif coffee_type == 'americano':
return Americano()
elif coffee_type == 'latte':
return Latte()
else:
return None
# 示例程序
if __name__ == '__main__':
coffee = CoffeeFactory.create_coffee('latte')
coffee = SyrupDecorator(coffee, 'vanilla')
coffee = MilkDecorator(coffee, 'almond')
coffee = FoamDecorator(coffee)
print(f'{coffee.get_description()} costs ${coffee.get_cost()}')
```
输出结果为:
```
Latte, vanilla syrup, almond milk, foam costs $4.39
```
相关推荐
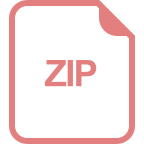
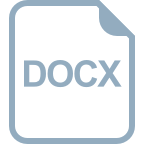
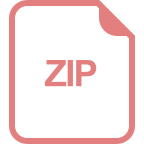














