声明一个异常类CException,它有成员函数Reason,它用来显示异常的类型,在子函数中触发异常,在主程序中处理异常,观察程序的执行流程,编写程序lab12_1.cpp。在CException类的成员函数Reason()中用cout显示异常的类型,在函数fn1()中用throw语句触发异常,在主函数的try模块中调用fn1(),在catch模块中捕获异常
时间: 2024-02-25 09:53:36 浏览: 87
好的,以下是相应的代码:
```c++
#include <iostream>
using namespace std;
class CException {
public:
CException(string reason) : m_reason(reason) {}
void Reason() { cout << "Exception: " << m_reason << endl; }
private:
string m_reason;
};
void fn1() {
throw CException("Error occurred in fn1()");
}
int main() {
try {
fn1();
}
catch (CException& e) {
e.Reason();
}
return 0;
}
```
程序的执行流程如下:
1. 程序开始执行,进入main函数;
2. 在try块中调用fn1函数;
3. fn1函数中使用throw语句抛出一个CException类型的异常,程序跳转到catch块中;
4. catch块中捕获到异常,并调用异常类CException的成员函数Reason()显示异常信息;
5. 程序结束。
希望这个例子对你有所帮助!
相关问题
声明一个异常类Cexception,有成员函数Reason(),用来显示异常的类型,在子函数中触发异常,在主程序中处理异常,观察程序的执行流程。
好的,下面是一个简单的实现:
```c++
#include <iostream>
using namespace std;
class Cexception {
public:
Cexception(string reason) : m_reason(reason) {}
void Reason() { cout << "Exception: " << m_reason << endl; }
private:
string m_reason;
};
void func() {
throw Cexception("Something went wrong!");
}
int main() {
try {
func();
}
catch(Cexception& e) {
e.Reason();
}
return 0;
}
```
在上面的代码中,我们定义了一个异常类 `Cexception`,它包含一个成员函数 `Reason()` 用于显示异常类型。在 `func()` 函数中,我们抛出了一个 `Cexception` 类型的异常。在主程序中,我们使用 `try-catch` 块来捕获异常,并调用 `Reason()` 函数来输出异常信息。
当程序运行时,因为 `func()` 函数抛出了一个异常,所以程序会进入 `catch` 块中,并输出异常信息:
```
Exception: Something went wrong!
```
这就是异常处理的基本流程。当程序遇到异常情况时,它会抛出一个异常,然后程序会在 `try` 块中寻找与该异常类型匹配的 `catch` 块,并执行其中的代码。如果找不到匹配的 `catch` 块,程序就会崩溃。
声明一个异常类CException,它有成员函数Reason,它用来显示异常的类型,在子函数中触发异常,在主程序中处理异常,观察程序的执行流程
好的,以下是一个简单的实现:
```c++
#include <iostream>
#include <string>
class CException {
public:
CException(const std::string& reason) : reason_(reason) {}
std::string Reason() const { return reason_; }
private:
std::string reason_;
};
int divide(int a, int b) {
if (b == 0) {
throw CException("Divide by zero!");
}
return a / b;
}
int main() {
int a, b;
std::cout << "Enter two integers: ";
std::cin >> a >> b;
try {
int result = divide(a, b);
std::cout << "Result: " << result << std::endl;
}
catch (const CException& e) {
std::cout << "Exception caught: " << e.Reason() << std::endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个异常类 `CException`,它有一个成员函数 `Reason`,用于返回异常的原因。然后,我们定义了一个 `divide` 函数,如果除数为零,则抛出一个 `CException` 异常。在主程序中,我们调用 `divide` 函数并捕获可能抛出的异常,如果出现异常,我们将异常的原因打印出来。如果没有异常,我们将除法的结果打印出来。
当我们运行这个程序并输入一个非零的除数时,它会输出除法的结果。如果我们输入零作为除数,它会抛出一个 `CException` 异常,并输出异常的原因。这个程序演示了如何在子函数中触发异常并在主程序中处理异常。
阅读全文
相关推荐
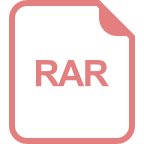
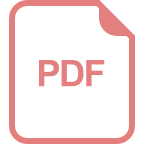












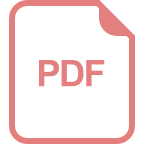