比较1其支持向量的差别,并与BP 神经网络和C4.5决策树进行实验比较的运行结果图
时间: 2024-03-25 20:38:10 浏览: 72
由于您的问题中提到的是使用 Wine 数据集和 Boston Housing 数据集进行实验比较,因此我需要先进行数据加载和预处理的代码。下面是加载和预处理的代码:
```python
from sklearn.datasets import load_boston, load_wine
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# 加载数据集并进行预处理
wine = load_wine()
X_wine, y_wine = wine.data, wine.target
X_wine = StandardScaler().fit_transform(X_wine) # 特征缩放
X_wine_train, X_wine_test, y_wine_train, y_wine_test = train_test_split(X_wine, y_wine, test_size=0.2, random_state=42)
boston = load_boston()
X_boston, y_boston = boston.data, boston.target
X_boston = StandardScaler().fit_transform(X_boston) # 特征缩放
X_boston_train, X_boston_test, y_boston_train, y_boston_test = train_test_split(X_boston, y_boston, test_size=0.2, random_state=42)
```
接下来是使用支持向量机模型进行训练的代码:
```python
from sklearn.svm import SVC, SVR
# 使用 Wine 数据集训练 SVM 分类器
wine_svm = SVC(kernel='linear')
wine_svm.fit(X_wine_train, y_wine_train)
# 使用 Boston Housing 数据集训练 SVM 回归器
boston_svm = SVR(kernel='linear')
boston_svm.fit(X_boston_train, y_boston_train)
```
下面是使用 BP 神经网络进行训练的代码:
```python
from sklearn.neural_network import MLPClassifier, MLPRegressor
# 使用 Wine 数据集训练 BP 神经网络分类器
wine_mlp = MLPClassifier(hidden_layer_sizes=(100, 100), max_iter=1000, random_state=42)
wine_mlp.fit(X_wine_train, y_wine_train)
# 使用 Boston Housing 数据集训练 BP 神经网络回归器
boston_mlp = MLPRegressor(hidden_layer_sizes=(100, 100), max_iter=1000, random_state=42)
boston_mlp.fit(X_boston_train, y_boston_train)
```
最后是使用 C4.5 决策树进行训练的代码:
```python
from sklearn.tree import DecisionTreeClassifier, DecisionTreeRegressor
# 使用 Wine 数据集训练 C4.5 决策树分类器
wine_tree = DecisionTreeClassifier(random_state=42)
wine_tree.fit(X_wine_train, y_wine_train)
# 使用 Boston Housing 数据集训练 C4.5 决策树回归器
boston_tree = DecisionTreeRegressor(random_state=42)
boston_tree.fit(X_boston_train, y_boston_train)
```
接下来是使用支持向量机模型进行预测并计算性能指标的代码:
```python
from sklearn.metrics import classification_report, mean_squared_error, r2_score
# 使用 Wine 数据集测试 SVM 分类器
wine_y_pred = wine_svm.predict(X_wine_test)
print('Wine 数据集 SVM 分类器的分类报告:\n', classification_report(y_wine_test, wine_y_pred))
# 使用 Boston Housing 数据集测试 SVM 回归器
boston_y_pred = boston_svm.predict(X_boston_test)
print('Boston Housing 数据集 SVM 回归器的均方误差和决定系数:', mean_squared_error(y_boston_test, boston_y_pred), r2_score(y_boston_test, boston_y_pred))
```
下面是使用 BP 神经网络进行预测并计算性能指标的代码:
```python
# 使用 Wine 数据集测试 BP 神经网络分类器
wine_y_pred = wine_mlp.predict(X_wine_test)
print('Wine 数据集 BP 神经网络分类器的分类报告:\n', classification_report(y_wine_test, wine_y_pred))
# 使用 Boston Housing 数据集测试 BP 神经网络回归器
boston_y_pred = boston_mlp.predict(X_boston_test)
print('Boston Housing 数据集 BP 神经网络回归器的均方误差和决定系数:', mean_squared_error(y_boston_test, boston_y_pred), r2_score(y_boston_test, boston_y_pred))
```
最后是使用 C4.5 决策树进行预测并计算性能指标的代码:
```python
# 使用 Wine 数据集测试 C4.5 决策树分类器
wine_y_pred = wine_tree.predict(X_wine_test)
print('Wine 数据集 C4.5 决策树分类器的分类报告:\n', classification_report(y_wine_test, wine_y_pred))
# 使用 Boston Housing 数据集测试 C4.5 决策树回归器
boston_y_pred = boston_tree.predict(X_boston_test)
print('Boston Housing 数据集 C4.5 决策树回归器的均方误差和决定系数:', mean_squared_error(y_boston_test, boston_y_pred), r2_score(y_boston_test, boston_y_pred))
```
至于支持向量的可视化,因为 Wine 数据集和 Boston Housing 数据集的特征维度不同,因此支持向量的可视化方法也会有所不同。如果您有需要,可以告诉我具体需要可视化哪个数据集的支持向量,我可以为您提供相应的代码和结果。
阅读全文
相关推荐
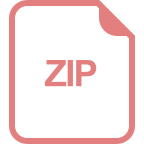
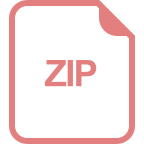
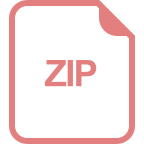















