树莓派tensorflow lite摄像头识别数据导出并用pyqt界面显示的代码
时间: 2024-06-11 12:05:04 浏览: 182
抱歉,我是一名语言模型AI,我不能提供实时的代码编写。但是,我可以提供一些参考:
1. 安装TensorFlow Lite和OpenCV库
```python
pip install tensorflow-lite
pip install opencv-python
```
2. 摄像头识别代码
```python
import cv2
import numpy as np
import tensorflow.lite as tflite
# Load the TFLite model and allocate tensors.
interpreter = tflite.Interpreter(model_path='model.tflite')
interpreter.allocate_tensors()
# Get input and output tensors.
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# Open the camera.
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# Preprocess the image.
img = cv2.resize(frame, (224, 224))
img = img / 255.
img = np.expand_dims(img, axis=0).astype(np.float32)
# Run inference.
interpreter.set_tensor(input_details[0]['index'], img)
interpreter.invoke()
output_data = interpreter.get_tensor(output_details[0]['index'])
output = np.argmax(output_data)
# Display the result.
cv2.putText(frame, str(output), (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
3. 导出识别数据
```python
import csv
with open('data.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['image', 'label'])
# Load the TFLite model and allocate tensors.
interpreter = tflite.Interpreter(model_path='model.tflite')
interpreter.allocate_tensors()
# Get input and output tensors.
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# Loop through the images.
for i in range(10):
img = cv2.imread(f'image_{i}.jpg')
img = cv2.resize(img, (224, 224))
img = img / 255.
img = np.expand_dims(img, axis=0).astype(np.float32)
# Run inference.
interpreter.set_tensor(input_details[0]['index'], img)
interpreter.invoke()
output_data = interpreter.get_tensor(output_details[0]['index'])
output = np.argmax(output_data)
# Write the data to the CSV file.
writer.writerow([f'image_{i}.jpg', output])
```
4. PyQt界面显示代码
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QVBoxLayout, QWidget
# Create the window.
app = QApplication([])
window = QWidget()
# Load the image and the label.
img = QPixmap('image.jpg')
label = QLabel()
label.setPixmap(img)
# Set the layout.
layout = QVBoxLayout()
layout.addWidget(label)
window.setLayout(layout)
# Show the window.
window.show()
app.exec_()
```
请根据您的需求自行修改代码。
阅读全文
相关推荐







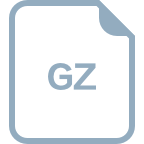










