poi-ooxml:3.8 删除莫一列
时间: 2023-08-15 09:06:41 浏览: 181
你可以使用Apache POI的XSSF工具类来实现删除某一列的操作。具体步骤如下:
1. 加载Excel文件并获取工作簿对象。
```java
// 读取Excel文件
FileInputStream fileInputStream = new FileInputStream("test.xlsx");
// 获取工作簿对象
XSSFWorkbook workbook = new XSSFWorkbook(fileInputStream);
```
2. 获取需要操作的工作表对象。
```java
// 获取第一个工作表
XSSFSheet sheet = workbook.getSheetAt(0);
```
3. 遍历需要删除的列,逐一删除单元格。
```java
// 需要删除的列号
int columnIndexToDelete = 2;
// 遍历所有行
for (Row row : sheet) {
// 获取当前行中需要删除的单元格
Cell cell = row.getCell(columnIndexToDelete);
if (cell != null) {
// 删除单元格
row.removeCell(cell);
}
// 将列号之后的单元格左移
for (int i = columnIndexToDelete + 1; i <= row.getLastCellNum(); i++) {
Cell currentCell = row.getCell(i);
if (currentCell != null) {
Cell newCell = row.createCell(i - 1, currentCell.getCellType());
newCell.setCellStyle(currentCell.getCellStyle());
switch (currentCell.getCellType()) {
case BOOLEAN:
newCell.setCellValue(currentCell.getBooleanCellValue());
break;
case NUMERIC:
newCell.setCellValue(currentCell.getNumericCellValue());
break;
case STRING:
newCell.setCellValue(currentCell.getStringCellValue());
break;
case FORMULA:
newCell.setCellFormula(currentCell.getCellFormula());
break;
default:
break;
}
row.removeCell(currentCell);
}
}
}
```
4. 将修改后的工作簿保存到文件中。
```java
FileOutputStream fileOutputStream = new FileOutputStream("test.xlsx");
workbook.write(fileOutputStream);
fileOutputStream.close();
```
完整代码如下:
```java
// 读取Excel文件
FileInputStream fileInputStream = new FileInputStream("test.xlsx");
// 获取工作簿对象
XSSFWorkbook workbook = new XSSFWorkbook(fileInputStream);
// 获取第一个工作表
XSSFSheet sheet = workbook.getSheetAt(0);
// 需要删除的列号
int columnIndexToDelete = 2;
// 遍历所有行
for (Row row : sheet) {
// 获取当前行中需要删除的单元格
Cell cell = row.getCell(columnIndexToDelete);
if (cell != null) {
// 删除单元格
row.removeCell(cell);
}
// 将列号之后的单元格左移
for (int i = columnIndexToDelete + 1; i <= row.getLastCellNum(); i++) {
Cell currentCell = row.getCell(i);
if (currentCell != null) {
Cell newCell = row.createCell(i - 1, currentCell.getCellType());
newCell.setCellStyle(currentCell.getCellStyle());
switch (currentCell.getCellType()) {
case BOOLEAN:
newCell.setCellValue(currentCell.getBooleanCellValue());
break;
case NUMERIC:
newCell.setCellValue(currentCell.getNumericCellValue());
break;
case STRING:
newCell.setCellValue(currentCell.getStringCellValue());
break;
case FORMULA:
newCell.setCellFormula(currentCell.getCellFormula());
break;
default:
break;
}
row.removeCell(currentCell);
}
}
}
// 保存修改后的工作簿到文件中
FileOutputStream fileOutputStream = new FileOutputStream("test.xlsx");
workbook.write(fileOutputStream);
fileOutputStream.close();
```
这里假设Excel文件中第一个工作表是需要操作的工作表,需要删除的列号为2(即第三列)。在遍历每一行时,如果当前行中存在需要删除的单元格,则使用`row.removeCell(cell)`方法删除该单元格。然后将列号之后的单元格左移一位,具体实现方法是先创建一个新的单元格,然后将原单元格的值拷贝到新单元格中,最后再使用`row.removeCell(currentCell)`方法删除原单元格。最后,将修改后的工作簿保存到文件中。
阅读全文
相关推荐












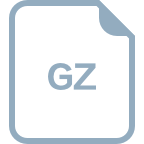




