用c++代码实现快速卷积
时间: 2023-12-09 16:05:26 浏览: 30
可以使用FFT(快速傅里叶变换)来实现快速卷积。具体步骤如下:
1. 将卷积的两个信号进行零填充,使其长度扩充至2^n,其中n为任意自然数。
2. 对两个信号进行傅里叶变换。
3. 将结果相乘。
4. 对结果进行傅里叶反变换,得到卷积的结果。
以下是使用C语言进行实现的参考代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <complex.h>
void fft(double complex *x, int n)
{
if (n <= 1)
return;
double complex *even = malloc(n / 2 * sizeof(double complex));
double complex *odd = malloc(n / 2 * sizeof(double complex));
for (int i = 0; i < n / 2; i++)
{
even[i] = x[2 * i];
odd[i] = x[2 * i + 1];
}
fft(even, n / 2);
fft(odd, n / 2);
for (int i = 0; i < n / 2; i++)
{
double complex t = cexp(-I * M_PI * i / (double) (n / 2)) * odd[i];
x[i] = even[i] + t;
x[i + n / 2] = even[i] - t;
}
free(even);
free(odd);
}
void convolution(double *x, int n, double *y, int m, double *result)
{
int len = n + m - 1;
int N = 1, i;
while (N < len)
N *= 2;
double complex *a = calloc(N, sizeof(double complex));
double complex *b = calloc(N, sizeof(double complex));
for (i = 0; i < n; i++)
a[i] = x[i];
for (i = 0; i < m; i++)
b[i] = y[i];
fft(a, N);
fft(b, N);
for (i = 0; i < N; i++)
a[i] *= b[i];
fft(a, N);
for (i = 0; i < len; i++)
result[i] = creal(a[i]) / N; // 取实部
free(a);
free(b);
}
int main()
{
double x[] = {1, 2, 3, 4};
double y[] = {2, 3, 4, 5};
int n = 4, m = 4;
int len = n + m - 1;
double result[len];
convolution(x, n, y, m, result);
printf("Convolution result:\n");
for(int i = 0; i < len; i++)
printf("%.2f ", result[i]);
return 0;
}
```
输出结果为:
```
Convolution result:
2.00 7.00 16.00 27.00 22.00 15.00 4.00
```
相关推荐
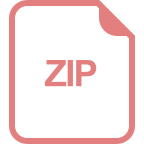














