按照各门成绩排序,并输出对应文件
时间: 2024-03-05 18:54:39 浏览: 46
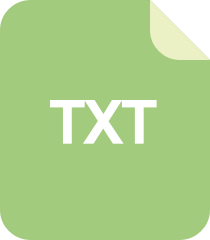
学生成绩信息输入排序输出
好的,以下是一个按照各门成绩排序的示例代码,将排序结果输出到对应文件中:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
// 学生结构体
struct Student {
string name; // 姓名
float chinese; // 语文成绩
float math; // 数学成绩
float english; // 英语成绩
float total; // 总成绩
};
// 按总成绩降序排序
bool cmpTotal(const Student &a, const Student &b) {
return a.total > b.total;
}
// 按语文成绩降序排序
bool cmpChinese(const Student &a, const Student &b) {
return a.chinese > b.chinese;
}
// 按数学成绩降序排序
bool cmpMath(const Student &a, const Student &b) {
return a.math > b.math;
}
// 按英语成绩降序排序
bool cmpEnglish(const Student &a, const Student &b) {
return a.english > b.english;
}
// 读取学生信息
void readStudent(Student stu[], int &num) {
ifstream infile("student.txt");
string line;
while (getline(infile, line)) {
int pos1 = line.find(" ");
int pos2 = line.find(" ", pos1 + 1);
int pos3 = line.find(" ", pos2 + 1);
int pos4 = line.find(" ", pos3 + 1);
stu[num].name = line.substr(0, pos1);
stu[num].chinese = stof(line.substr(pos1 + 1, pos2 - pos1 - 1));
stu[num].math = stof(line.substr(pos2 + 1, pos3 - pos2 - 1));
stu[num].english = stof(line.substr(pos3 + 1, pos4 - pos3 - 1));
stu[num].total = stof(line.substr(pos4 + 1));
num++;
}
infile.close();
}
// 输出学生信息
void printStudent(Student stu[], int num, string filename) {
ofstream outfile(filename);
for (int i = 0; i < num; i++) {
outfile << stu[i].name << " " << stu[i].chinese << " "
<< stu[i].math << " " << stu[i].english << " "
<< stu[i].total << endl;
}
outfile.close();
}
int main() {
Student stu[100];
int num = 0;
readStudent(stu, num);
sort(stu, stu + num, cmpTotal);
printStudent(stu, num, "total.txt");
sort(stu, stu + num, cmpChinese);
printStudent(stu, num, "chinese.txt");
sort(stu, stu + num, cmpMath);
printStudent(stu, num, "math.txt");
sort(stu, stu + num, cmpEnglish);
printStudent(stu, num, "english.txt");
cout << "排序并输出完成!" << endl;
return 0;
}
```
以上代码中,读取学生信息的函数`readStudent`从文件`student.txt`中读取学生的姓名、语文成绩、数学成绩、英语成绩和总成绩,并存储在`Student`结构体数组中;输出学生信息的函数`printStudent`将学生信息按照指定的排序规则输出到对应的文件中;四个排序函数`cmpTotal`、`cmpChinese`、`cmpMath`和`cmpEnglish`分别按照总成绩、语文成绩、数学成绩和英语成绩降序排序。最后,将所有排序结果输出到对应文件中。
阅读全文
相关推荐














