qml实现左边导航列表,右边文本输入区
时间: 2023-07-18 09:44:30 浏览: 128
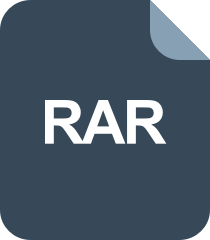
jQuery左侧列表动态导航代码
实现这个功能可以使用Qt Quick中的ListView和TextArea组件。
首先,我们需要定义一个数据模型(ListModel),用于存储左边导航列表的数据。假设我们要显示三个导航项,可以这样定义数据模型:
```
ListModel {
id: listModel
ListElement { title: "导航1" }
ListElement { title: "导航2" }
ListElement { title: "导航3" }
}
```
接下来,在界面中添加一个ListView组件,用于显示导航列表。我们需要设置ListView的model属性为上面定义的数据模型,并设置delegate属性为一个Item组件,用于定义每个列表项的外观。代码如下:
```
ListView {
id: listView
model: listModel
delegate: Item {
height: 40
Text {
text: title
font.pixelSize: 16
color: listView.currentIndex === index ? "white" : "black"
}
MouseArea {
anchors.fill: parent
onClicked: listView.currentIndex = index
}
}
}
```
在上面的代码中,我们使用Text组件显示每个列表项的标题,并根据当前选中项的索引来设置文本颜色。另外,我们还添加了一个MouseArea组件,用于处理列表项的点击事件。当用户点击某个列表项时,我们将当前选中项的索引设置为点击项的索引。
接下来,我们需要在界面中添加一个TextArea组件,用于显示右边的文本输入区。代码如下:
```
TextArea {
id: textArea
anchors.top: parent.top
anchors.left: listView.right
anchors.right: parent.right
anchors.bottom: parent.bottom
readOnly: true
}
```
在上面的代码中,我们将TextArea组件的左边界与ListView组件的右边界对齐,从而实现左边导航列表,右边文本输入区的布局。我们将TextArea的readOnly属性设置为true,表示文本输入区是只读的。
最后,我们需要在ListView的onCurrentIndexChanged事件中更新TextArea的文本内容。代码如下:
```
ListView {
// ...
onCurrentIndexChanged: {
if (currentIndex >= 0 && currentIndex < listModel.count) {
var title = listModel.get(currentIndex).title
textArea.text = "当前选中项:" + title
} else {
textArea.text = ""
}
}
}
```
在上面的代码中,我们判断当前选中项的索引是否在数据模型范围内。如果是,则获取当前选中项的标题,并在TextArea中显示。如果选中项的索引超出范围,则清空TextArea的文本内容。
完整的代码示例如下:
```
import QtQuick 2.0
ListModel {
id: listModel
ListElement { title: "导航1" }
ListElement { title: "导航2" }
ListElement { title: "导航3" }
}
Rectangle {
width: 600
height: 400
ListView {
id: listView
width: 200
model: listModel
delegate: Item {
height: 40
Text {
text: title
font.pixelSize: 16
color: listView.currentIndex === index ? "white" : "black"
}
MouseArea {
anchors.fill: parent
onClicked: listView.currentIndex = index
}
}
onCurrentIndexChanged: {
if (currentIndex >= 0 && currentIndex < listModel.count) {
var title = listModel.get(currentIndex).title
textArea.text = "当前选中项:" + title
} else {
textArea.text = ""
}
}
}
TextArea {
id: textArea
anchors.top: parent.top
anchors.left: listView.right
anchors.right: parent.right
anchors.bottom: parent.bottom
readOnly: true
}
}
```
阅读全文
相关推荐
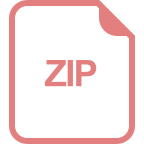
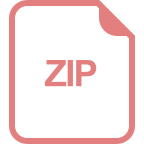
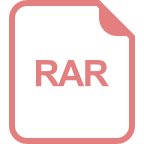
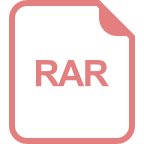
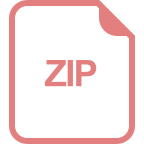
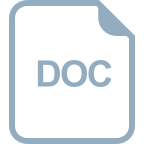
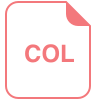
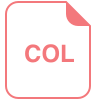

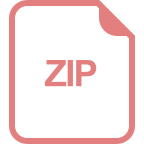
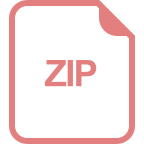
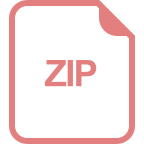
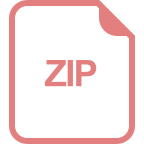
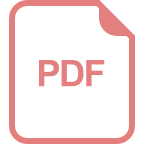
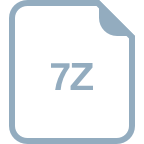
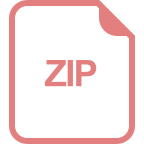
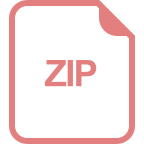
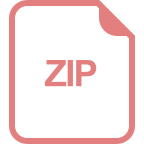