写一个C#俄罗斯方块游戏
时间: 2023-10-19 11:13:27 浏览: 144
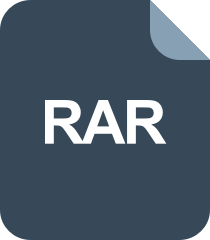
C#编的俄罗斯方块游戏
很高兴回答您的问题!以下是一个简单的C#俄罗斯方块游戏的示例代码:
```csharp
using System;
using System.Collections.Generic;
namespace Tetris
{
class Program
{
static void Main(string[] args)
{
// 初始化游戏板和方块
int[,] board = new int[20, 10];
Block currentBlock = new Block();
// 游戏循环
while (true)
{
// 显示游戏板和当前方块
DisplayBoard(board, currentBlock);
// 获取用户输入
ConsoleKeyInfo keyInfo = Console.ReadKey(true);
// 移动方块或旋转方块
if (keyInfo.Key == ConsoleKey.LeftArrow)
{
currentBlock.MoveLeft(board);
}
else if (keyInfo.Key == ConsoleKey.RightArrow)
{
currentBlock.MoveRight(board);
}
else if (keyInfo.Key == ConsoleKey.DownArrow)
{
currentBlock.MoveDown(board);
}
else if (keyInfo.Key == ConsoleKey.UpArrow)
{
currentBlock.Rotate(board);
}
// 下落方块
if (!currentBlock.MoveDown(board))
{
// 如果方块无法再下落,将方块固定到游戏板上
currentBlock.Lock(board);
// 判断是否有一行已经填满
int linesCleared = 0;
for (int i = board.GetLength(0) - 1; i >= 0; i--)
{
bool isFullLine = true;
for (int j = 0; j < board.GetLength(1); j++)
{
if (board[i, j] == 0)
{
isFullLine = false;
break;
}
}
if (isFullLine)
{
// 如果有一行已经填满,将该行删除并将上面的方块下移
for (int k = i; k > 0; k--)
{
for (int j = 0; j < board.GetLength(1); j++)
{
board[k, j] = board[k - 1, j];
}
}
linesCleared++;
i++; // 由于上面的方块下移,需要重新检查该行
}
}
// 如果有行被删除,更新分数
if (linesCleared > 0)
{
UpdateScore(linesCleared);
}
// 创建一个新的方块
currentBlock = new Block();
}
}
}
static void DisplayBoard(int[,] board, Block currentBlock)
{
// 清空控制台
Console.Clear();
// 显示分数
Console.WriteLine("Score: " + score);
// 显示游戏板和当前方块
for (int i = 0; i < board.GetLength(0); i++)
{
for (int j = 0; j < board.GetLength(1); j++)
{
if (board[i, j] == 0)
{
Console.Write(".");
}
else
{
Console.Write("X");
}
}
Console.Write(" ");
for (int j = 0; j < 4; j++)
{
for (int k = 0; k < 4; k++)
{
if (currentBlock.Shape[j, k] == 0)
{
Console.Write(".");
}
else
{
Console.Write("X");
}
}
Console.WriteLine();
}
}
}
static int score = 0;
static void UpdateScore(int linesCleared)
{
score += linesCleared * 100;
}
}
class Block
{
public int[,] Shape { get; private set; }
public int Row { get; private set; }
public int Col { get; private set; }
private static Random random = new Random();
private static List<int[,]> shapes = new List<int[,]>()
{
new int[,] { { 1, 1 }, { 1, 1 } },
new int[,] { { 0, 1, 0 }, { 1, 1, 1 } },
new int[,] { { 1, 0, 0 }, { 1, 1, 1 } },
new int[,] { { 0, 0, 1 }, { 1, 1, 1 } },
new int[,] { { 1, 1, 0 }, { 0, 1, 1 } },
new int[,] { { 0, 1, 1 }, { 1, 1, 0 } },
new int[,] { { 1, 1, 1, 1 } }
};
public Block()
{
// 随机选择一个方块
int index = random.Next(shapes.Count);
Shape = shapes[index];
// 将方块放到游戏板的顶部中央
Row = 0;
Col = (10 - Shape.GetLength(1)) / 2;
}
public bool MoveLeft(int[,] board)
{
if (Col > 0 && CanMove(board, Row, Col - 1))
{
Col--;
return true;
}
return false;
}
public bool MoveRight(int[,] board)
{
if (Col < board.GetLength(1) - Shape.GetLength(1) && CanMove(board, Row, Col + 1))
{
Col++;
return true;
}
return false;
}
public bool MoveDown(int[,] board)
{
if (Row < board.GetLength(0) - Shape.GetLength(0) && CanMove(board, Row + 1, Col))
{
Row++;
return true;
}
return false;
}
public void Rotate(int[,] board)
{
int[,] rotatedShape = new int[Shape.GetLength(1), Shape.GetLength(0)];
for (int i = 0; i < Shape.GetLength(0); i++)
{
for (int j = 0; j < Shape.GetLength(1); j++)
{
rotatedShape[j, Shape.GetLength(0) - 1 - i] = Shape[i, j];
}
}
if (CanMove(board, Row, Col, rotatedShape))
{
Shape = rotatedShape;
}
}
public void Lock(int[,] board)
{
for (int i = 0; i < Shape.GetLength(0); i++)
{
for (int j = 0; j < Shape.GetLength(1); j++)
{
if (Shape[i, j] != 0)
{
board[Row + i, Col + j] = 1;
}
}
}
}
private bool CanMove(int[,] board, int newRow, int newCol)
{
return CanMove(board, newRow, newCol, Shape);
}
private bool CanMove(int[,] board, int newRow, int newCol, int[,] newShape)
{
for (int i = 0; i < newShape.GetLength(0); i++)
{
for (int j = 0; j < newShape.GetLength(1); j++)
{
if (newShape[i, j] != 0)
{
int row = newRow + i;
int col = newCol + j;
if (row < 0 || row >= board.GetLength(0) || col < 0 || col >= board.GetLength(1) || board[row, col] != 0)
{
return false;
}
}
}
}
return true;
}
}
}
```
此代码创建了一个简单的俄罗斯方块游戏,其中方块可以左右移动、向下落、旋转,并且可以与游戏板上的方块进行碰撞检测。该代码可以运行在控制台中。
阅读全文
相关推荐
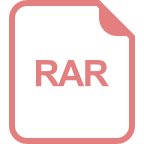
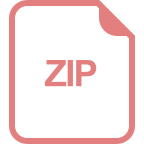
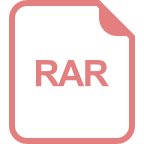
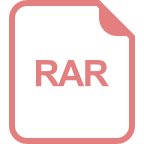
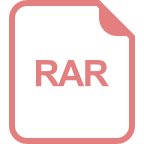
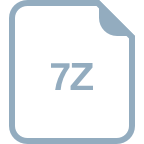
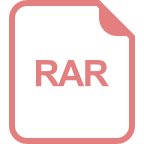
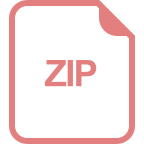
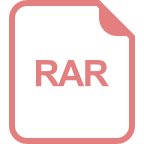
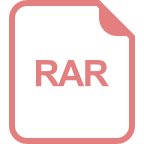
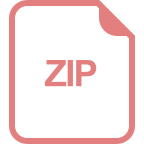
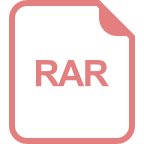
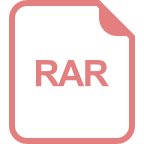