pytorch演示一下,反向传播的过程
时间: 2023-06-30 15:15:46 浏览: 68
好的,我可以给您演示一下 PyTorch 中反向传播的基本过程。
假设有一个简单的神经网络模型:
```python
import torch
class Net(torch.nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = torch.nn.Linear(10, 5)
self.fc2 = torch.nn.Linear(5, 2)
def forward(self, x):
x = torch.nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
```
其中,该模型包含两个线性层,输入层大小为 10,输出层大小为 2。
现在,我们可以使用 PyTorch 实现反向传播的过程,来更新每个参数的梯度。
```python
# 定义损失函数
criterion = torch.nn.MSELoss()
# 定义优化器
optimizer = torch.optim.SGD(net.parameters(), lr=0.01)
# 训练模型
for epoch in range(100):
# 前向传播
outputs = net(inputs)
loss = criterion(outputs, targets)
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
在这个例子中,我们使用了均方误差损失函数(MSE),并使用随机梯度下降(SGD)优化器来更新模型参数。在训练过程中,我们通过前向传播计算模型输出和损失值,然后通过反向传播计算每个参数的梯度,并使用优化器来更新参数。
希望这个例子能够帮助您更好地理解 PyTorch 中反向传播的基本过程。
相关推荐
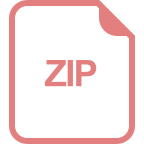














