opencv调用yolov7模型结构图
时间: 2023-11-09 21:07:10 浏览: 45
以下是使用OpenCV调用YoloV7模型的基本结构图:
1. 加载YoloV7模型:使用OpenCV的dnn模块加载YoloV7模型的权重文件和配置文件。
2. 准备输入图像:将待识别的图像读入内存,并且进行预处理,如缩放、裁剪等操作。
3. 对输入图像进行推理:将预处理后的图像输入到模型中,进行推理操作,得到目标检测结果。
4. 解析输出结果:将模型输出的结果进行解析,得到检测到的目标的位置、类别、置信度等信息。
5. 绘制检测结果:使用OpenCV的绘图函数,将检测结果绘制在原图上,并显示出来。
6. 释放内存:释放使用的内存空间。
下面是一个简单的代码示例:
```python
import cv2
# 加载YoloV7模型
net = cv2.dnn.readNetFromDarknet("yolov7.cfg", "yolov7.weights")
# 准备输入图像
img = cv2.imread("test.jpg")
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 对输入图像进行推理
net.setInput(blob)
outputs = net.forward(net.getUnconnectedOutLayersNames())
# 解析输出结果
boxes = []
confidences = []
classIds = []
for output in outputs:
for detection in output:
scores = detection[5:]
classId = np.argmax(scores)
confidence = scores[classId]
if confidence > confThreshold:
box = detection[0:4] * np.array([img.shape[1], img.shape[0], img.shape[1], img.shape[0]])
centerX, centerY, width, height = box.astype("int")
x, y = int(centerX - (width / 2)), int(centerY - (height / 2))
boxes.append([x, y, int(width), int(height)])
confidences.append(float(confidence))
classIds.append(classId)
# 绘制检测结果
indices = cv2.dnn.NMSBoxes(boxes, confidences, confThreshold, nmsThreshold)
for i in indices:
i = i[0]
box = boxes[i]
x, y, w, h = box
label = str(classes[classIds[i]])
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示检测结果
cv2.imshow("Detection result", img)
cv2.waitKey(0)
# 释放内存
cv2.destroyAllWindows()
```
相关推荐
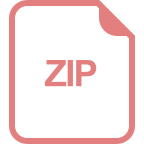
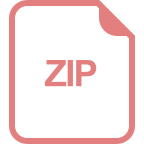














