请用c语言链表编写一个学生成绩统计系统,能过依据录入的学生成绩进行由大到小排序
时间: 2024-01-22 21:20:24 浏览: 70
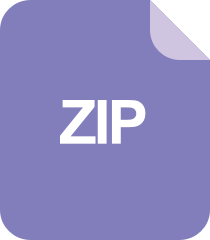
纯C语言实现学生成绩管理系统,采用链表结构

以下是一个简单的学生成绩统计系统,使用链表来存储学生信息和成绩,并按照成绩由大到小的顺序进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
struct student {
char name[20];
int score;
struct student *next;
};
void insert(struct student **head, char name[], int score) {
struct student *new_node = (struct student*)malloc(sizeof(struct student));
if (new_node == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
strcpy(new_node->name, name);
new_node->score = score;
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
} else {
struct student *curr = *head;
struct student *prev = NULL;
while (curr != NULL && curr->score > score) {
prev = curr;
curr = curr->next;
}
if (prev == NULL) {
new_node->next = *head;
*head = new_node;
} else {
new_node->next = curr;
prev->next = new_node;
}
}
}
void display(struct student *head) {
printf("Name\tScore\n");
printf("-----------------\n");
struct student *curr = head;
while (curr != NULL) {
printf("%s\t%d\n", curr->name, curr->score);
curr = curr->next;
}
}
int main() {
struct student *head = NULL;
int n, score;
char name[20];
printf("Enter the number of students: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter the name and score of student %d: ", i+1);
scanf("%s %d", name, &score);
insert(&head, name, score);
}
display(head);
return 0;
}
```
在此代码中,我们定义了一个结构体 `student`,其中包含学生姓名和成绩,并使用链表来存储学生信息。`insert()` 函数用于在链表中插入新的节点,按照成绩由大到小的顺序进行排序。`display()` 函数用于显示链表中所有节点的信息。在 `main()` 函数中,我们首先要求用户输入学生数量,然后循环读取每个学生的姓名和成绩,并将其插入链表中。最后,我们调用 `display()` 函数来显示所有学生的信息,按照成绩由大到小的顺序进行排序。
阅读全文
相关推荐

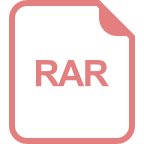
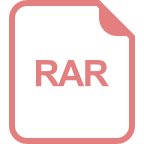
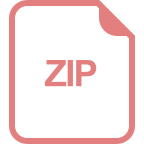
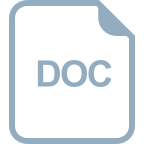


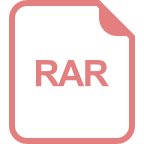
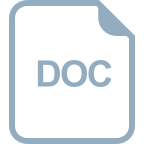
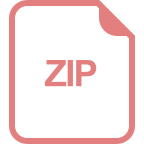
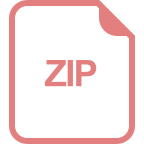
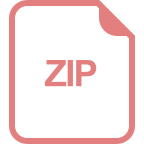
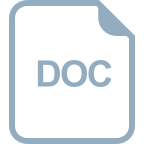