用类c描述C语言完成链表节点的定义和初始化、查找、销毁等基本操作,再将(3,6,8,9)和(2,5,8,10,12,16)两个非递减的有序链表合并为一个非递增的有序链表,仍然用原来的存储空间,并且要有每步操作的注释。
时间: 2023-08-31 17:28:29 浏览: 84
### 回答1:
链表节点的定义:
```c
struct Node {
int data;
struct Node *next;
};
```
链表节点的初始化:
```c
// 创建一个新的节点并返回指针
struct Node* createNode(int data) {
struct Node *node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
return node;
}
```
链表的查找:
```c
// 在链表中查找值为data的节点并返回指针
struct Node* findNode(struct Node *head, int data) {
struct Node *p = head;
while (p != NULL && p->data != data) {
p = p->next;
}
return p;
}
```
链表的销毁:
```c
// 销毁整个链表
void destroyList(struct Node *head) {
struct Node *p = head;
while (p != NULL) {
struct Node *q = p->next;
free(p);
p = q;
}
}
```
合并两个有序链表:
```c
// 将两个有序链表head1和head2合并成一个非递增的有序链表
struct Node* merge(struct Node *head1, struct Node *head2) {
// 如果有一个链表为空,则直接返回另一个链表
if (head1 == NULL) {
return head2;
}
if (head2 == NULL) {
return head1;
}
// 定义指针p1和p2分别指向链表head1和head2的第一个节点
struct Node *p1 = head1;
struct Node *p2 = head2;
// 定义指针p和newHead分别指向新链表的第一个节点
struct Node *newHead = NULL;
struct Node *p = NULL;
// 循环比较p1和p2节点的值,并将较小的节点插入到新链表中
while (p1 != NULL && p2 != NULL) {
if (p1->data <= p2->data) {
// 插入p1节点
if (newHead == NULL) {
newHead = p1;
p = p1;
} else {
p->next = p1;
p = p->next;
}
p1 = p1->next;
} else {
// 插入p2节点
if (newHead == NULL) {
newHead = p2;
p = p2;
} else {
p->next = p2;
p = p->next;
}
p2 = p2->next;
}
}
// 将剩余节点插入到新链表中
while (p1 != NULL) {
p->next = p1;
p = p->next;
p1 = p1->next;
}
while (p2 != NULL) {
p->next = p2;
p = p->next;
p2 = p2->next;
}
// 反转新链表,变成非递增的有序链表
struct Node *prev = NULL;
p = newHead;
while (p != NULL) {
struct Node *next = p->next;
p->next = prev;
prev = p;
p = next;
}
return prev;
}
```
完整的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点的定义
struct Node {
int data;
struct Node *next;
};
// 创建一个新的节点并返回指针
struct Node* createNode(int data) {
struct Node *node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
return node;
}
// 在链表中查找值为data的节点并返回指针
struct Node* findNode(struct Node *head, int data) {
struct Node *p = head;
while (p != NULL && p->data != data) {
p = p->next;
}
return p;
}
// 销毁整个链表
void destroyList(struct Node *head) {
struct Node *p = head;
while (p != NULL) {
struct Node *q = p->next;
free(p);
p = q;
}
}
// 将两个有序链表head1和head2合并成一个非递增的有序链表
struct Node* merge(struct Node *head1, struct Node *head2) {
// 如果有一个链表为空,则直接返回另一个链表
if (head1 == NULL) {
return head2;
}
if (head2 == NULL) {
return head1;
}
// 定义指针p1和p2分别指向链表head1和head2的第一个节点
struct Node *p1 = head1;
struct Node *p2 = head2;
// 定义指针p和newHead分别指向新链表的第一个节点
struct Node *newHead = NULL;
struct Node *p = NULL;
// 循环比较p1和p2节点的值,并将较小的节点插入到新链表中
while (p1 != NULL && p2 != NULL) {
if (p1->data <= p2->data) {
// 插入p1节点
if (newHead == NULL) {
newHead = p1;
p = p1;
} else {
p->next = p1;
p = p->next;
}
p1 = p1->next;
} else {
// 插入p2节点
if (newHead == NULL) {
newHead = p2;
p = p2;
} else {
p->next = p2;
p = p->next;
}
p2 = p2->next;
}
}
// 将剩余节点插入到新链表中
while (p1 != NULL) {
p->next = p1;
p = p->next;
p1 = p1->next;
}
while (p2 != NULL) {
p->next = p2;
p = p->next;
p2 = p2->next;
}
// 反转新链表,变成非递增的有序链表
struct Node *prev = NULL;
p = newHead;
while (p != NULL) {
struct Node *next = p->next;
p->next = prev;
prev = p;
p = next;
}
return prev;
}
int main() {
// 创建链表1
struct Node *head1 = createNode(3);
head1->next = createNode(6);
head1->next->next = createNode(8);
head1->next->next->next = createNode(9);
// 创建链表2
struct Node *head2 = createNode(2);
head2->next = createNode(5);
head2->next->next = createNode(8);
head2->next->next->next = createNode(10);
head2->next->next->next->next = createNode(12);
head2->next->next->next->next->next = createNode(16);
// 合并链表1和链表2
struct Node *newHead = merge(head1, head2);
// 输出合并后的链表
printf("合并后的链表:");
struct Node *p = newHead;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
// 释放内存
destroyList(head1);
destroyList(head2);
destroyList(newHead);
return 0;
}
```
输出结果为:
```
合并后的链表:16 12 10 9 8 8 6 5 3 2
```
### 回答2:
链表节点的定义和初始化如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 初始化链表节点
ListNode* createNode(int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
```
链表的查找和销毁操作如下:
```c
// 查找链表中的值为target的节点,并返回指向该节点的指针
ListNode* searchNode(ListNode* head, int target) {
if (head == NULL) {
return NULL;
}
ListNode* current = head;
while (current != NULL) {
if (current->data == target) {
return current;
}
current = current->next;
}
return NULL;
}
// 销毁链表,释放节点占用的内存空间
void destroyList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
ListNode* temp = current;
current = current->next;
free(temp);
}
}
```
合并两个非递减的有序链表为一个非递增的有序链表如下:
```c
// 合并两个非递减的有序链表为一个非递增的有序链表
ListNode* mergeLists(ListNode* list1, ListNode* list2) {
if (list1 == NULL) {
return list2;
}
if (list2 == NULL) {
return list1;
}
ListNode* newList = NULL;
ListNode* current = NULL;
while (list1 != NULL && list2 != NULL) {
// 比较两个链表当前节点的值
if (list1->data <= list2->data) {
// 将list1当前节点移动到新链表中
if (newList == NULL) {
newList = list1;
current = newList;
} else {
current->next = list1;
current = current->next;
}
list1 = list1->next;
} else {
// 将list2当前节点移动到新链表中
if (newList == NULL) {
newList = list2;
current = newList;
} else {
current->next = list2;
current = current->next;
}
list2 = list2->next;
}
}
// 如果list1或list2还有剩余节点,则将其直接连接到新链表末尾
if (list1 != NULL) {
current->next = list1;
}
if (list2 != NULL) {
current->next = list2;
}
// 反转新链表,使其变为非递增有序链表
ListNode* prev = NULL;
ListNode* next = NULL;
current = newList;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
```
以上是使用类c描述C语言完成链表节点的定义和初始化、查找、销毁等基本操作,以及将两个非递减的有序链表合并为一个非递增的有序链表的过程。每个操作都有相应的注释解释其功能。
阅读全文
相关推荐
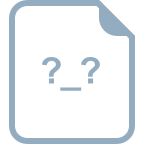
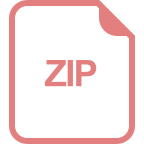


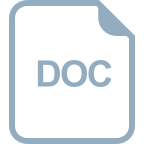
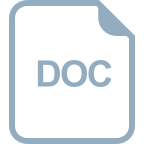

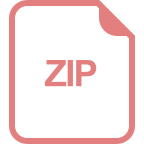
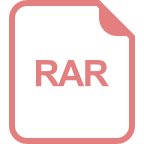
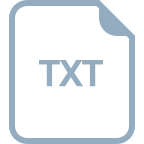
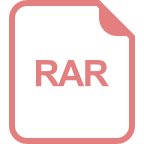
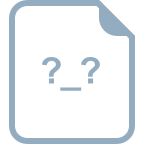
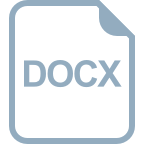
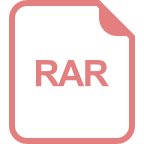
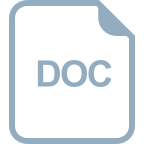
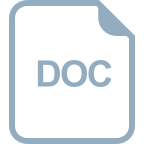
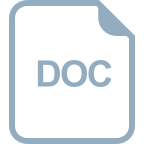
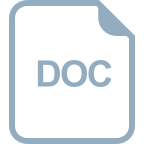