CSharp 实现Cruskal算法 源代码
时间: 2024-05-12 09:18:08 浏览: 118
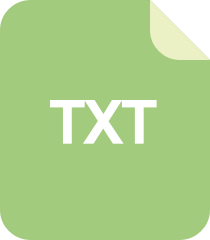
kruskal算法实现

可以直接回答,以下是CSharp实现Cruskal算法的示例代码:
```csharp
using System;
using System.Collections.Generic;
public class Graph
{
public int V, E;
public List<Edge> edges;
public Graph(int v, int e)
{
V = v;
E = e;
edges = new List<Edge>(E);
for (int i = 0; i < E; ++i)
edges.Add(new Edge());
}
public int Find(int[] parent, int i)
{
if (parent[i] == -1)
return i;
return Find(parent, parent[i]);
}
public void Union(int[] parent, int x, int y)
{
int xset = Find(parent, x);
int yset = Find(parent, y);
parent[xset] = yset;
}
public void KruskalMST()
{
Edge[] result = new Edge[V];
int e = 0;
int i = 0;
edges.Sort();
int[] parent = new int[V];
for (int j = 0; j < V; ++j)
parent[j] = -1;
while (e < V - 1)
{
Edge next_edge = edges[i++];
int x = Find(parent, next_edge.src);
int y = Find(parent, next_edge.dest);
if (x != y)
{
result[e++] = next_edge;
Union(parent, x, y);
}
}
Console.WriteLine("Following are the edges in " +
"the constructed MST");
for (i = 0; i < e; ++i)
Console.WriteLine(result[i].src + " - " +
result[i].dest + ": " + result[i].weight);
}
}
public class Edge : IComparable<Edge>
{
public int src, dest, weight;
public int CompareTo(Edge other)
{
return weight.CompareTo(other.weight);
}
}
public class Test
{
public static void Main()
{
int V = 4;
int E = 5;
Graph graph = new Graph(V, E);
graph.edges[0].src = 0;
graph.edges[0].dest = 1;
graph.edges[0].weight = 10;
graph.edges[1].src = 0;
graph.edges[1].dest = 2;
graph.edges[1].weight = 6;
graph.edges[2].src = 0;
graph.edges[2].dest = 3;
graph.edges[2].weight = 5;
graph.edges[3].src = 1;
graph.edges[3].dest = 3;
graph.edges[3].weight = 15;
graph.edges[4].src = 2;
graph.edges[4].dest = 3;
graph.edges[4].weight = 4;
graph.KruskalMST();
}
}
```
希望能对你有所帮助!接下来,请问你想问我什么问题?
阅读全文
相关推荐
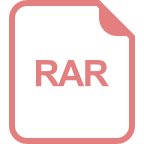
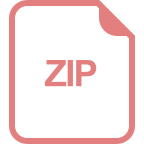
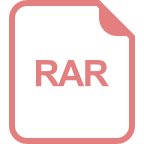
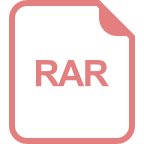
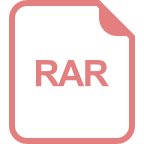
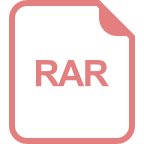
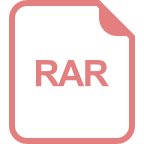
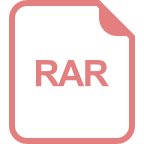
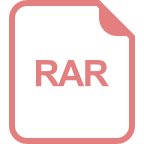
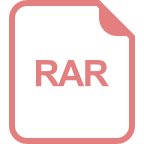
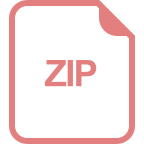
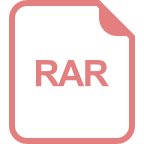
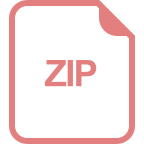
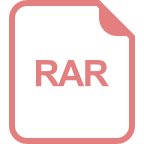