设置了ping和server 发现还是app处于后台就断开了
时间: 2024-01-24 15:02:48 浏览: 77
即使您设置了ping和pong消息保持WebSocket连接活动状态,仍然可能会出现WebSocket连接断开的问题。这可能是由于Android系统内存管理策略导致的,当您的应用程序处于后台状态时,系统可能会关闭一些正在运行的进程以释放更多的内存资源,从而导致WebSocket连接断开。
为了解决这个问题,您可以尝试使用Android的Foreground Service来保持WebSocket连接处于活动状态。Foreground Service是一种前台服务,它会在系统状态栏中显示一个持续的通知,以告知用户该服务正在运行。
以下是一个简单的WebSocket Foreground Service示例:
```java
public class WebSocketService extends Service {
private static final String CHANNEL_ID = "WebSocketServiceChannel";
private WebSocketClient mWebSocketClient;
@Override
public void onCreate() {
super.onCreate();
createNotificationChannel();
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
startWebSocket();
startForeground(1, createNotification());
return START_STICKY;
}
private void startWebSocket() {
URI uri;
try {
uri = new URI("ws://your-websocket-server-url");
} catch (URISyntaxException e) {
e.printStackTrace();
return;
}
mWebSocketClient = new WebSocketClient(uri) {
@Override
public void onOpen(ServerHandshake handshakedata) {
// WebSocket连接已打开
}
@Override
public void onMessage(String message) {
// 收到WebSocket消息
}
@Override
public void onClose(int code, String reason, boolean remote) {
// WebSocket连接已关闭,尝试重新连接
try {
Thread.sleep(5000); // 等待5秒后重新连接
} catch (InterruptedException e) {
e.printStackTrace();
}
connect();
}
@Override
public void onError(Exception ex) {
// WebSocket连接出错
}
};
mWebSocketClient.setPingInterval(5000); // 设置ping消息发送间隔
mWebSocketClient.setPongInterval(10000); // 设置pong消息接收超时时间
mWebSocketClient.connect();
}
private Notification createNotification() {
Intent notificationIntent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0);
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_launcher_background)
.setContentTitle("WebSocketService")
.setContentText("WebSocketService is running")
.setContentIntent(pendingIntent)
.setPriority(NotificationCompat.PRIORITY_DEFAULT);
return builder.build();
}
private void createNotificationChannel() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, "WebSocketServiceChannel", NotificationManager.IMPORTANCE_DEFAULT);
NotificationManager manager = getSystemService(NotificationManager.class);
manager.createNotificationChannel(channel);
}
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
在这个示例中,我们创建了一个Foreground Service来保持WebSocket连接处于活动状态。在WebSocketService的onStartCommand方法中,我们启动WebSocket连接并使用startForeground方法将该服务设置为前台服务,并在状态栏中显示一个通知。在WebSocket连接断开时,我们在onClose方法中重新连接WebSocket服务器。
请注意,为了在Android 8.0及以上版本上显示通知,您需要创建一个通知通道。在我们的示例中,我们在createNotificationChannel方法中创建了一个通知通道。
最后,您可以在应用程序的MainActivity中启动WebSocketService:
```java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
startService(new Intent(this, WebSocketService.class));
}
}
```
这个示例只是一个基本的WebSocket Foreground Service实现,您需要根据您的具体需求进行修改和优化。同时,您还需要考虑更多的细节和错误处理,以确保WebSocket连接的稳定性和可靠性。
阅读全文
相关推荐









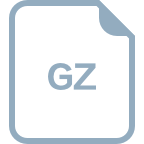

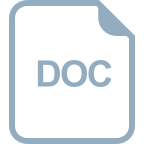

