用vue生成一个公告栏
时间: 2024-05-10 22:16:35 浏览: 152
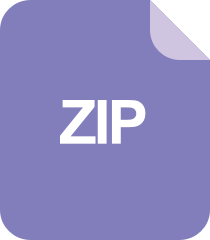
公告栏
好的,以下是用 Vue.js 生成一个简单的公告栏的示例代码:
1. 首先,在你的 Vue 项目中创建一个组件,例如 NoticeBoard.vue:
```
<template>
<div class="notice-board">
<h2>{{ title }}</h2>
<ul>
<li v-for="notice in notices" :key="notice.id">{{ notice.content }}</li>
</ul>
</div>
</template>
<script>
export default {
name: 'NoticeBoard',
props: {
title: {
type: String,
default: 'Notice Board'
},
notices: {
type: Array,
required: true
}
}
}
</script>
<style scoped>
.notice-board {
border: 1px solid #ccc;
padding: 10px;
margin: 10px 0;
}
.notice-board h2 {
margin-top: 0;
}
.notice-board ul {
list-style: none;
margin: 0;
padding: 0;
}
.notice-board li {
margin: 5px 0;
}
</style>
```
2. 在你的父组件中使用 NoticeBoard 组件,并传入所需的数据:
```
<template>
<div>
<NoticeBoard :notices="notices" />
</div>
</template>
<script>
import NoticeBoard from './NoticeBoard.vue'
export default {
name: 'App',
components: {
NoticeBoard
},
data() {
return {
notices: [
{
id: 1,
content: 'Notice 1'
},
{
id: 2,
content: 'Notice 2'
},
{
id: 3,
content: 'Notice 3'
}
]
}
}
}
</script>
```
在这个示例中,我们创建了一个名为 NoticeBoard 的组件,该组件接受两个 props:title 和 notices。title 是公告板的标题,notices 是一个包含公告内容的数组。在组件内部,我们使用了 v-for 指令来循环遍历 notices 数组,并将每个公告的内容渲染为一个 li 元素。
在父组件中,我们引入了 NoticeBoard 组件,并传入了一个包含三个公告的数组作为 notices prop 的值。这样,当我们渲染父组件时,NoticeBoard 组件会被渲染出来,并显示出三个公告的内容。
希望这个示例可以帮助你理解如何使用 Vue.js 创建一个简单的公告栏。
阅读全文
相关推荐
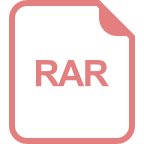
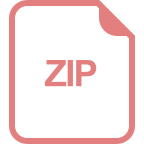
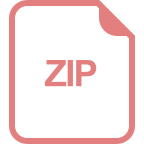
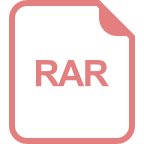
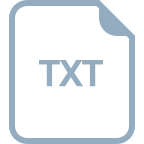
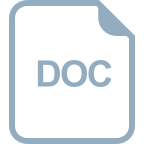
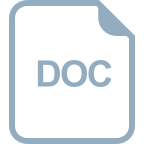
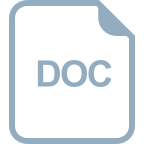
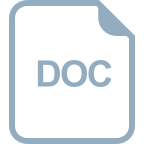
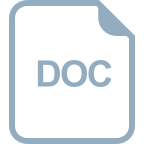

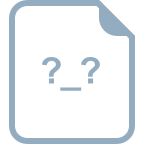