遍历文件夹中的文件,给每个文档第一行的末尾加一个;
时间: 2024-04-29 12:25:46 浏览: 71
以下是Python代码实现:
```python
import os
folder_path = "path/to/folder" # 文件夹路径
for filename in os.listdir(folder_path): # 遍历文件夹中的文件
if filename.endswith(".txt"): # 如果是txt文件
file_path = os.path.join(folder_path, filename) # 获取文件路径
with open(file_path, "r+") as file: # 以读写模式打开文件
first_line = file.readline() # 获取第一行的内容
if not first_line.endswith(";"): # 如果末尾没有分号
file.seek(0) # 将光标移到文件开头
file.write(first_line.rstrip() + ";") # 在末尾加上分号
file.write("\n") # 写入换行符
```
代码解析:
1. 首先定义文件夹路径 `folder_path`。
2. 使用 `os.listdir()` 函数遍历文件夹中的文件,获取文件名 `filename`。
3. 判断文件是否为txt文件,可以使用 `filename.endswith(".txt")` 来判断。
4. 如果是txt文件,使用 `os.path.join()` 函数获取文件路径 `file_path`。
5. 使用 `with open(file_path, "r+") as file` 打开文件,以读写模式打开文件,这样可以同时读取和修改文件。
6. 使用 `file.readline()` 函数获取第一行的内容 `first_line`。
7. 判断第一行的末尾是否已经有分号,如果没有分号,使用 `file.seek(0)` 函数将光标移到文件开头,使用 `file.write()` 函数在第一行末尾加上分号,并在行末写入换行符。
8. 由于使用了 `with` 上下文管理器,所以文件会自动关闭。
阅读全文
相关推荐

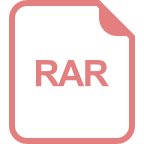

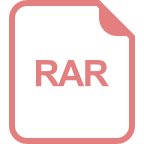




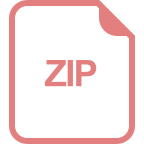

