mybatis-plus生成集成pgsql
时间: 2023-07-04 16:06:24 浏览: 55
要在 MyBatis-Plus 中使用 PostgreSQL,需要在项目中添加相应的依赖和配置。
1. 添加依赖
在项目 `pom.xml` 文件中添加以下依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.9</version>
</dependency>
```
其中,`mybatis-plus-boot-starter` 是 MyBatis-Plus 的启动器依赖,`postgresql` 是 PostgreSQL 的 JDBC 驱动。
2. 配置数据源
在 `application.yml` 文件中配置 PostgreSQL 的数据源:
```yaml
spring:
datasource:
url: jdbc:postgresql://localhost:5432/db_name
username: username
password: password
driver-class-name: org.postgresql.Driver
```
其中,`url`、`username` 和 `password` 分别是 PostgreSQL 数据库的连接信息。
3. 配置 MyBatis-Plus
在项目中创建一个 `MybatisPlusConfig` 配置类,用于配置 MyBatis-Plus:
```java
@Configuration
public class MybatisPlusConfig {
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
return interceptor;
}
@Bean
public PaginationInterceptor paginationInterceptor() {
return new PaginationInterceptor();
}
@Bean
public ISqlInjector sqlInjector() {
return new LogicSqlInjector();
}
@Bean
public ConfigurationCustomizer configurationCustomizer() {
return configuration -> configuration.setUseDeprecatedExecutor(false);
}
}
```
其中,`MybatisPlusInterceptor` 和 `PaginationInterceptor` 分别是 MyBatis-Plus 的拦截器,`ISqlInjector` 是 SQL 注入器,`ConfigurationCustomizer` 是 MyBatis 的配置定制器。
4. 生成实体类和 Mapper
使用 MyBatis-Plus 的代码生成器可以快速生成实体类和 Mapper。
在项目中添加以下依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.3.2</version>
</dependency>
```
在 `resources` 目录下创建一个 `generator` 文件夹,并在其中创建一个 `generatorConfig.xml` 配置文件,用于配置代码生成器:
```xml
<generatorConfiguration>
<context id="pgsqlTables" targetRuntime="MyBatis3">
<jdbcConnection driverClass="org.postgresql.Driver" connectionURL="jdbc:postgresql://localhost:5432/db_name" userId="username" password="password">
</jdbcConnection>
<javaModelGenerator targetPackage="com.example.demo.entity" targetProject="src/main/java">
<property name="enableSubPackages" value="true" />
<property name="trimStrings" value="true" />
</javaModelGenerator>
<sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources">
<property name="enableSubPackages" value="true" />
</sqlMapGenerator>
<javaClientGenerator type="XMLMAPPER" targetPackage="com.example.demo.mapper" targetProject="src/main/java">
<property name="enableSubPackages" value="true" />
</javaClientGenerator>
<table tableName="user" domainObjectName="User" />
</context>
</generatorConfiguration>
```
其中,`jdbcConnection` 配置 PostgreSQL 的连接信息,`javaModelGenerator` 配置生成实体类的包名和路径,`sqlMapGenerator` 配置生成 Mapper 的包名和路径,`javaClientGenerator` 配置生成 Mapper 接口的包名和路径,`table` 指定要生成的表名和实体类名。
运行以下命令即可生成代码:
```bash
mvn mybatis-plus:generator
```
5. 使用 MyBatis-Plus 进行操作
在项目中使用 MyBatis-Plus 进行操作 PostgreSQL 数据库的数据。
例如,使用 `BaseMapper` 的 `selectList` 方法查询所有用户信息:
```java
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Override
public List<User> list() {
return baseMapper.selectList(null);
}
}
```
其中,`UserMapper` 是自动生成的 Mapper 接口,`User` 是自动生成的实体类。`baseMapper` 是 MyBatis-Plus 提供的基础 Mapper,包含了一些常用的 CRUD 操作方法。`selectList` 方法可以查询所有用户信息。
相关推荐
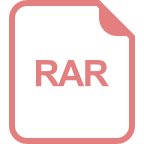
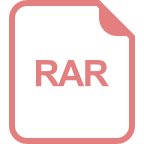
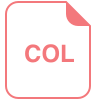
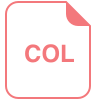
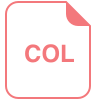
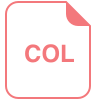
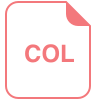








