写遗传算法优化支持向量机的代码
时间: 2023-08-11 21:53:43 浏览: 74
好的,以下是一个简单的遗传算法优化支持向量机的Python代码示例:
```python
import random
import numpy as np
from sklearn.svm import SVC
# 定义适应度函数
def fitness(individual, X_train, y_train):
# 解码个体
C = individual[0]
gamma = individual[1]
kernel = individual[2]
# 构建SVM分类器
clf = SVC(C=C, gamma=gamma, kernel=kernel)
# 训练分类器并计算准确率
clf.fit(X_train, y_train)
accuracy = clf.score(X_train, y_train)
return accuracy
# 定义遗传算法参数
POPULATION_SIZE = 50
NUM_GENERATIONS = 100
CROSSOVER_RATE = 0.8
MUTATION_RATE = 0.1
# 定义搜索空间
C_RANGE = (0.1, 100)
GAMMA_RANGE = (0.0001, 10)
KERNELS = ['linear', 'poly', 'rbf', 'sigmoid']
# 初始化种群
population = []
for i in range(POPULATION_SIZE):
C = random.uniform(*C_RANGE)
gamma = random.uniform(*GAMMA_RANGE)
kernel = random.choice(KERNELS)
individual = [C, gamma, kernel]
population.append(individual)
# 遗传算法主循环
for generation in range(NUM_GENERATIONS):
# 计算适应度
fitness_scores = []
for individual in population:
fitness_score = fitness(individual, X_train, y_train)
fitness_scores.append(fitness_score)
# 选择
selected_population = []
for i in range(POPULATION_SIZE):
# 轮盘赌选择
probabilities = np.array(fitness_scores) / sum(fitness_scores)
index = np.random.choice(range(POPULATION_SIZE), p=probabilities)
selected_population.append(population[index])
# 交叉
crossover_population = []
for i in range(POPULATION_SIZE):
if random.random() < CROSSOVER_RATE:
# 随机选择两个个体进行交叉
parent1 = random.choice(selected_population)
parent2 = random.choice(selected_population)
child1 = parent1.copy()
child2 = parent2.copy()
# 随机选择一个基因进行交叉
gene_index = random.randint(0, 2)
child1[gene_index], child2[gene_index] = child2[gene_index], child1[gene_index]
crossover_population += [child1, child2]
else:
crossover_population.append(selected_population[i])
# 变异
mutation_population = []
for i in range(POPULATION_SIZE):
if random.random() < MUTATION_RATE:
# 随机选择一个个体进行变异
parent = random.choice(crossover_population)
child = parent.copy()
# 随机选择一个基因进行变异
gene_index = random.randint(0, 2)
if gene_index == 0:
child[gene_index] += random.choice([-1, 1]) * random.uniform(0, 10)
elif gene_index == 1:
child[gene_index] += random.choice([-1, 1]) * random.uniform(0, 1)
else:
child[gene_index] = random.choice(KERNELS)
mutation_population.append(child)
else:
mutation_population.append(crossover_population[i])
# 更新种群
population = mutation_population
# 在测试集上评估最佳个体
best_individual = max(population, key=lambda x: fitness(x, X_train, y_train))
C = best_individual[0]
gamma = best_individual[1]
kernel = best_individual[2]
clf = SVC(C=C, gamma=gamma, kernel=kernel)
clf.fit(X_train, y_train)
test_accuracy = clf.score(X_test, y_test)
print('Test accuracy: %.2f%%' % (test_accuracy * 100))
```
需要注意的是,这只是一个简单的示例,实际的遗传算法优化支持向量机可能需要更复杂的适应度函数、选择、交叉和变异操作等。此外,还需要对搜索空间进行合理的选择和设置,以及对遗传算法的参数进行调优。
阅读全文
相关推荐








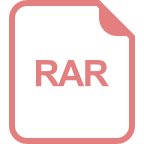








