用java写一个发送post的请求
时间: 2023-07-01 16:12:18 浏览: 46
可以使用Java的HttpURLConnection类来实现发送POST请求,以下是一个简单的示例代码:
```java
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class HttpUtil {
public static String sendPostRequest(String url, String params) {
HttpURLConnection connection = null;
BufferedWriter writer = null;
BufferedReader reader = null;
try {
// 创建URL对象
URL requestUrl = new URL(url);
// 打开连接
connection = (HttpURLConnection) requestUrl.openConnection();
// 设置请求方法
connection.setRequestMethod("POST");
// 设置连接超时时间
connection.setConnectTimeout(5000);
// 设置读取超时时间
connection.setReadTimeout(5000);
// 设置是否向httpUrlConnection输出,因为post请求参数要放在http正文内,所以需要设为true
connection.setDoOutput(true);
// 设置是否从httpUrlConnection读入,默认情况下是true
connection.setDoInput(true);
// 设置请求头
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded; charset=UTF-8");
// 建立连接
connection.connect();
// 获取输出流
writer = new BufferedWriter(new OutputStreamWriter(connection.getOutputStream(), StandardCharsets.UTF_8));
// 发送请求参数
writer.write(params);
// 清空输出流
writer.flush();
// 获取输入流
reader = new BufferedReader(new InputStreamReader(connection.getInputStream(), StandardCharsets.UTF_8));
// 读取返回结果
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
return response.toString();
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭连接
try {
if (writer != null) {
writer.close();
}
if (reader != null) {
reader.close();
}
if (connection != null) {
connection.disconnect();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
}
```
以上代码使用HttpURLConnection类发送POST请求,需要传入请求的URL和请求参数。在发送POST请求时,需要设置请求方法为POST,并且将请求参数放在请求正文中。请求头中需要设置Content-Type为application/x-www-form-urlencoded,这是POST请求常用的一种ContentType。发送请求时需要注意设置超时时间、获取输入输出流、清空输出流等操作。
相关推荐
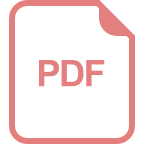
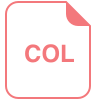
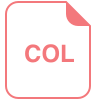
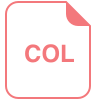











