Online test questions: search, extract, and process data from a list
时间: 2024-02-22 16:56:47 浏览: 67
I apologize for misunderstanding your question earlier. Here is an example implementation in Python to search, extract, and process data from a list:
```
# Example list of dictionaries
data = [
{'name': 'Alice', 'age': 25, 'gender': 'F'},
{'name': 'Bob', 'age': 30, 'gender': 'M'},
{'name': 'Charlie', 'age': 35, 'gender': 'M'},
{'name': 'David', 'age': 40, 'gender': 'M'},
{'name': 'Eve', 'age': 45, 'gender': 'F'}
]
# Search for all males in the list
males = [d for d in data if d['gender'] == 'M']
# Extract the names of all females in the list and store in a new list
female_names = [d['name'] for d in data if d['gender'] == 'F']
# Process the ages of all individuals in the list and compute the average age
ages = [d['age'] for d in data]
average_age = sum(ages) / len(ages)
# Print the results
print('Males in the list:', males)
print('Names of females in the list:', female_names)
print('Average age of individuals in the list:', average_age)
```
This example uses a list of dictionaries to represent data, but the same concepts can be applied to other types of data structures. The code searches for a specific gender, extracts names, and processes ages to compute the average age.
阅读全文
相关推荐
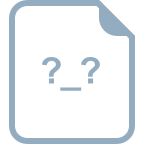
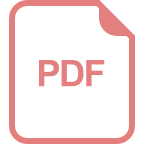
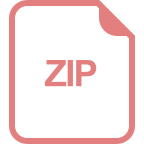
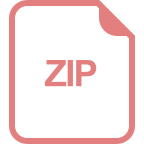
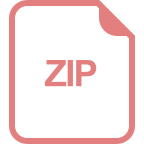
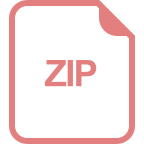
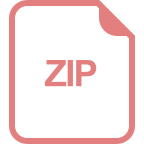
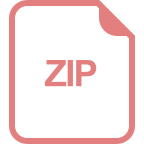
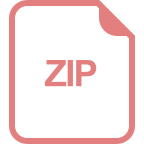
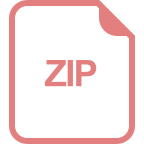
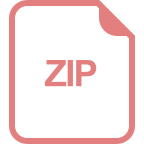
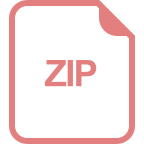
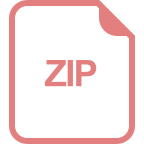
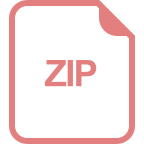
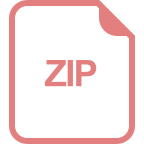
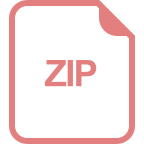
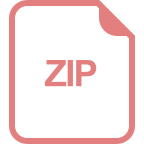
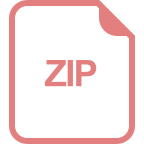
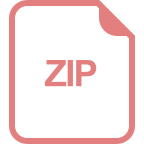