开发一个winpcap的嗅探器
时间: 2023-09-24 08:09:51 浏览: 95
要开发一个WinPcap的嗅探器,可以按照以下步骤进行:
1. 下载并安装WinPcap开发包:在WinPcap的官网上下载WinPcap开发包,并按照安装向导完成安装。
2. 创建一个新的WinPcap项目:在Visual Studio中创建一个新的WinPcap项目,并将WinPcap库添加到项目中。
3. 编写代码:使用WinPcap提供的API函数来实现数据包的嗅探和数据包分析功能。例如,可以使用pcap_open_live函数打开一个网络接口,使用pcap_loop函数来循环读取数据包并进行处理,使用pcap_sendpacket函数发送数据包,等等。
4. 编译和调试:将代码编译为可执行文件,并使用调试工具进行调试,以确保代码的正确性和稳定性。
以下是一个简单的WinPcap嗅探器示例代码,可以作为参考:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <pcap.h>
void packet_handler(u_char *param, const struct pcap_pkthdr *header, const u_char *pkt_data)
{
printf("Packet Received!\n");
}
int main()
{
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t *handle;
struct bpf_program fp;
char filter_exp[] = "ip";
bpf_u_int32 net, mask;
// Get the default network interface
if (pcap_lookupnet("eth0", &net, &mask, errbuf) == -1)
{
printf("Error getting network interface.\n");
return 1;
}
// Open the network interface in promiscuous mode
handle = pcap_open_live("eth0", 65535, 1, 1000, errbuf);
if (handle == NULL)
{
printf("Error opening network interface: %s\n", errbuf);
return 1;
}
// Compile the filter expression
if (pcap_compile(handle, &fp, filter_exp, 0, net) == -1)
{
printf("Error compiling filter expression: %s\n", pcap_geterr(handle));
pcap_close(handle);
return 1;
}
// Apply the filter expression
if (pcap_setfilter(handle, &fp) == -1)
{
printf("Error setting filter expression: %s\n", pcap_geterr(handle));
pcap_close(handle);
return 1;
}
// Start capturing packets
pcap_loop(handle, -1, packet_handler, NULL);
// Close the network interface
pcap_close(handle);
return 0;
}
```
这段代码打开了名为“eth0”的网络接口,编译了一个过滤表达式“ip”,并使用pcap_loop函数循环读取数据包并调用packet_handler函数进行处理。在packet_handler函数中,简单地打印了一条消息表示收到了一个数据包。
相关推荐
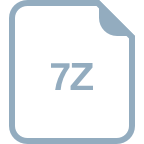







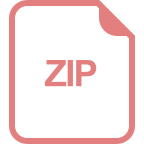
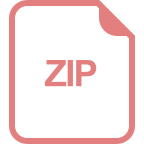
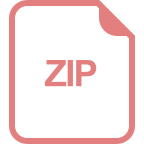
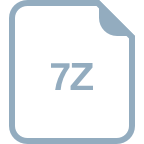
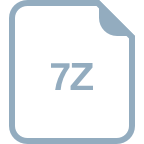