batch_size = x.size(0) tlist=[] for i in range(x.size(0)): y = x[i, :, :, :] y = y.permute(1, 2, 0) y = y.squeeze(2) y = y.cpu().numpy() img_norm_int = (y * 255).astype(np.uint8) img_255 = img_norm_int / img_norm_int.max() * 255 y = img_255.astype(np.uint8) lines = self.lsd.detect(cv2.convertScaleAbs(y))[0] if len(lines)<5: lines=cut_segments(lines, 5) lines=torch.tensor(lines) lines = np.array(lines) indices = np.arange(len(lines)) np.random.shuffle(indices) indices = indices[:5] lines = lines[indices] lines = torch.from_numpy(lines) if lines.shape == [5,1,4]: lines = torch.squeeze(lines,dim=1) tlist.append(lines) x = torch.stack(tlist, dim=0)
时间: 2024-02-14 08:21:32 浏览: 27
这段代码的作用是对输入的 `x` 张量进行预处理,并且返回一个新的张量 `x`,用于后续的处理。具体来说,它首先对输入的 `x` 张量进行循环,对每一个样本进行处理。对于每一个样本,它会进行以下步骤:
1. 将张量的第一维作为 batch_size,即 `batch_size = x.size(0)`。
2. 将张量沿着第一个维度进行循环,即 `for i in range(x.size(0)):`。
3. 将输入的 `x` 张量的第一个维度(batch_size)去掉,保留后面的三个维度作为一张图片的尺寸。
4. 对于每张图片,先将通道维度移到最后一个维度上,即 `y = y.permute(1, 2, 0)`。
5. 去掉通道维度,即 `y = y.squeeze(2)`。
6. 将图片转换为 numpy 数组,即 `y = y.cpu().numpy()`。
7. 对数组进行归一化,并转换为 uint8 类型,即 `img_norm_int = (y * 255).astype(np.uint8)`。
8. 将归一化后的数组进行缩放到 0-255 的范围内,即 `img_255 = img_norm_int / img_norm_int.max() * 255`。
9. 将缩放后的数组转换为 uint8 类型,即 `y = img_255.astype(np.uint8)`。
10. 通过 LSD 算法检测出图片中的线段,即 `lines = self.lsd.detect(cv2.convertScaleAbs(y))[0]`。
11. 判断检测出的线段是否小于 5 条,如果小于 5 条,则进行截取(即 `lines=cut_segments(lines, 5)`),补齐为 5 条,并转换为张量(即 `lines=torch.tensor(lines)`)。
12. 将线段转换为 numpy 数组,随机选择其中的 5 条线段(即 `indices = np.arange(len(lines))`、`np.random.shuffle(indices)`、`indices = indices[:5]`、`lines = lines[indices]`),并将其转换为张量(即 `lines = torch.from_numpy(lines)`)。
13. 如果线段的形状为 `[5,1,4]`,则将其压缩为 `[5,4]`。否则,不做处理(即 `if lines.shape == [5,1,4]:`、`lines = torch.squeeze(lines,dim=1)`)。
14. 将处理后的线段张量添加到 `tlist` 列表中(即 `tlist.append(lines)`)。
15. 将处理后的线段张量列表 `tlist` 堆叠成一个新的张量 `x`,并作为函数的返回值,即 `x = torch.stack(tlist, dim=0)`。
如果您有其他问题,可以继续提出。
相关推荐
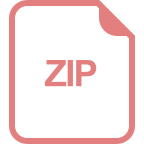
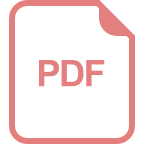














