帮我写一段提取word表格数据的代码
时间: 2024-05-08 13:19:40 浏览: 168
好的,以下是提取Word表格数据的Python代码示例:
```python
import docx
#打开Word文件
doc = docx.Document('document.docx')
#获取第一个表格
table = doc.tables[0]
#遍历表格每一行
for i, row in enumerate(table.rows):
#遍历每一列
for j, cell in enumerate(row.cells):
#获取单元格内容
cell_text = cell.text
print('第{}行第{}列的内容是:{}'.format(i+1, j+1, cell_text))
```
这段代码可以打开名为`document.docx`的Word文件,并提取出第一个表格的所有数据。你可以根据需要修改代码来适应不同的数据提取需求。
相关问题
帮我写一段整行提取word表格数据的代码
以下是利用Python中的openpyxl库提取Word表格数据的示例代码:
```python
import openpyxl
from docx import Document
# 打开Word文档
doc = Document('example.docx')
# 定位到指定表格
table = doc.tables[0]
# 创建Excel工作簿
workbook = openpyxl.Workbook()
# 获取活动的工作表
worksheet = workbook.active
# 遍历表格的所有行和列
for i, row in enumerate(table.rows):
for j, cell in enumerate(row.cells):
# 提取单元格的文本内容
text = cell.text
# 将数据写入Excel工作表中的单元格
worksheet.cell(row=i+1, column=j+1, value=text)
# 保存Excel工作簿
workbook.save('example.xlsx')
```
此代码可以将Word表格中的数据提取并保存到Excel文件中,但需要注意的是,代码仅供参考,具体实现可根据实际情况进行修改和调整。
帮我写一段爬取word文档中表格内容为“得分:”的下一格内容
### 回答1:
答案:可以使用Python的docx模块来实现这一功能,具体代码如下:from docx import Documentdocument = Document('test.docx')for table in document.tables:
for row in table.rows:
for cell in row.cells:
if '得分:' in cell.text:
print(cell.next_sibling.text)
### 回答2:
对于爬取Word文档中表格内容为“得分:”的下一格内容,你可以使用Python的第三方库python-docx来进行操作。以下是一个简单的示例代码:
```python
from docx import Document
def crawl_table_content(filename):
doc = Document(filename)
table = doc.tables[0] # 假设表格在文档的第一个位置
for row in table.rows:
for cell in row.cells:
if cell.text == '得分:':
next_cell_index = cell._tc.getnext().get('gridSpan', 1) - 1
next_cell = row.cells[row.cells.index(cell) + next_cell_index]
return next_cell.text
return None # 如果没有找到指定内容,返回None
# 调用示例
filename = '你的Word文档路径'
result = crawl_table_content(filename)
if result is not None:
print(result)
else:
print("未找到目标内容")
```
以上示例代码中,首先使用python-docx库的Document类加载Word文档。然后,我们假设目标表格位于文档的第一个位置,并遍历表格中的每一个单元格。当找到文本为“得分:”的单元格时,我们可以通过cell对象的_tc属性获取下一个单元格的索引,并使用index方法在行单元格列表中找到该单元格。最后,我们返回该下一格单元格的文本内容。
请注意,这只是一个简单的示例,具体实现可能会因为Word文档的格式和表格结构而有所不同。你可以根据需求进行适当的修改和定制。
### 回答3:
要实现爬取word文档中表格内容为“得分:”的下一格内容,可以使用Python的python-docx库进行操作。
首先,需要安装python-docx库。在终端中运行以下命令来安装:
```
pip install python-docx
```
然后,在Python脚本中导入所需的库:
```python
from docx import Document
```
接下来,使用open()函数打开word文档:
```python
doc = Document('文件路径/文件名.docx')
```
然后,通过遍历所有段落和表格的方式来查找目标内容:
```python
for paragraph in doc.paragraphs:
if '得分:' in paragraph.text:
table = paragraph.tables[0] # 假设表格在该段落的下一行
cell_value = table.cell(0, 1).text # 第一行第二列的单元格
print(cell_value)
break
```
最后,可以将找到的目标内容进行处理或保存:
```python
with open('输出文件路径/输出文件名.txt', 'w', encoding='utf-8') as file:
file.write(cell_value)
```
以上就是使用python-docx库来爬取word文档中表格内容为“得分:”的下一格内容的方法。通过遍历文档中的段落和表格,找到目标内容并进行提取,然后进行处理或保存。
阅读全文
相关推荐
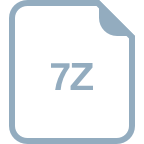
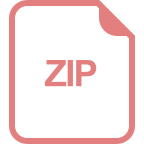
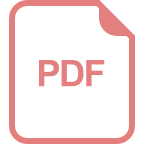
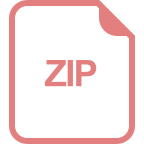
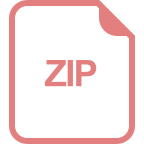
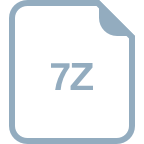
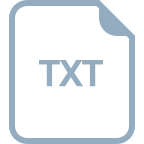
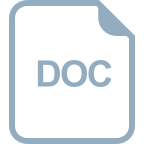






