ArrayRef<uint8_t> arr(code, nbyte)
时间: 2024-03-21 18:39:36 浏览: 94
`ArrayRef<uint8_t> arr(code, nbyte)`表示创建一个名为`arr`的`ArrayRef`对象,它引用了一个长度为`nbyte`的字节数组,数组的起始地址为`code`。这个类模板中的类型参数`uint8_t`表示数组中元素的类型。
举个例子,假设我们有一个字节数组`code`,它的长度为`nbyte`,我们可以使用以下代码来创建一个`ArrayRef`对象:
```
#include "llvm/ADT/ArrayRef.h"
#include <cstdint>
using namespace llvm;
void foo(uint8_t *code, int nbyte) {
ArrayRef<uint8_t> arr(code, nbyte);
// 对数组进行操作
for (auto i : arr) {
// ...
}
}
```
在上面的代码中,我们定义了一个函数`foo`,它接受一个指向字节数组`code`的指针和数组的长度`nbyte`。在函数中,我们通过`ArrayRef<uint8_t> arr(code, nbyte)`的方式创建了一个`ArrayRef`对象`arr`,它引用了`code`指向的字节数组。我们可以通过`for`循环遍历数组中的每个元素,并进行相应的操作。
相关问题
#include<string> #include"resource.h" #include<opencv2/opencv.hpp> #include<opencv2/core.hpp> #include <zxing/DecodeHints.h> #include <zxing/MultiFormatReader.h> #include <zxing/Result.h> #include <zxing/BinaryBitmap.h> #include <zxing/common/GlobalHistogramBinarizer.h> using namespace zxing; using namespace std; class OpenCVLuminanceSource : public zxing::LuminanceSource { private: cv::Mat image_; public: OpenCVLuminanceSource(cv::Mat image) : LuminanceSource(image.cols, image.rows), image_(image) {} zxing::ArrayRef<char> getRow(int y, zxing::ArrayRef<char> row) const { int width = getWidth(); if (!row || row->size() < width) { row = zxing::ArrayRef<char>(width); } const uchar* imgRow = image_.ptr<uchar>(y); memcpy(&row[0], imgRow, width); return row; } zxing::ArrayRef<char> getMatrix() const { int width = getWidth(); int height = getHeight(); zxing::ArrayRef<char> matrix = zxing::ArrayRef<char>(width * height); for (int y = 0; y < height; ++y) { const uchar* imgRow = image_.ptr<uchar>(y); memcpy(&matrix[y * width], imgRow, width); } return matrix; } }; int main() { cv::Mat image = cv::imread("path/to/your/image.jpg", cv::IMREAD_GRAYSCALE); cv::Mat image = cv::imread("path/to/your/image.jpg", cv::IMREAD_GRAYSCALE); OpenCVLuminanceSource source(image); zxing::Ref<zxing::LuminanceSource> luminanceSource(&source); zxing::Ref<zxing::Binarizer> binarizer = zxing::Binarizer::createBinarizer(luminanceSource); zxing::Ref<zxing::BinaryBitmap> bitmap = zxing::Ref<zxing::BinaryBitmap>(new zxing::BinaryBitmap(binarizer)); zxing::DecodeHints hints; hints.setTryHarder(true); zxing::MultiFormatReader reader; zxing::Ref<zxing::Result> result = reader.decode(bitmap, hints); std::string decodedData = result->getText()->getText(); std::cout << "Decoded data: " << decodedData << std::endl; }
您的代码看起来是使用 OpenCV 和 zxing 库来对图像进行解码。请注意,您在代码中有两次定义 `cv::Mat image`,请删除其中一次,以避免重复定义的错误。
此外,您还需要确保已正确引入 OpenCV 和 zxing 库,并在编译时链接到这些库。
如果您仍然遇到错误,请提供完整的错误信息和上下文,以便我可以更好地帮助您解决问题。
bool runTransform(Node* n, Graph& graph, NodeDestroyType& destroy_current) override { ostringstream os; string strBoundingBoxSizeName; Node *node = n->inputs()[0]->node()->inputs()[0]->node(); Node *mul1 = node; Node *pow = node->outputs()[0]->uses()[0].user; Node *mul2 = node->outputs()[0]->uses()[0].user->outputs()[0]->uses()[0].user; Value *tmp = mul1->inputs()[0]->node()->outputs()[0]; ArrayRef<Value *> target_confidence(tmp); Node *newNode = graph.create(Symbol(kBoundingBoxSize), target_confidence); // creat anchor grid Value *anchorGrid; createAnchorGridCoeff(graph, mul1, mul2, &anchorGrid); newNode->addInput(anchorGrid); mNumber++; os << "bounding_box_size_"<<mNumber; strBoundingBoxSizeName = os.str(); newNode->outputs()[0]->setSizes(mul2->outputs()[0]->sizes()); newNode->outputs()[0]->setUniqueName(strBoundingBoxSizeName); newNode->insertBefore(mul1); mul2->replaceAllUsesWith(newNode); pow->replaceAllUsesWith(newNode); pow->destroy(); mul1->replaceAllUsesWith(newNode); mul1->destroy(); destroy_current = NodeDestroyType::DestroyOne; return true; }这段代码什么意思
这段代码是一个函数,函数名为 runTransform。该函数的作用是在给定的Graph中对输入的Node n 进行一些转换操作,并返回一个布尔值。
具体来说,该函数首先获取输入Node n 的第一个输入的第一个输入的Node,将其赋值给node。然后,获取node的输出的第一个使用者,将其赋值给pow。再获取pow的输出的第一个使用者的输出的第一个使用者,将其赋值给mul2。接下来,获取mul1的输入的第一个Node的输出的第一个Value,将其存储在名为target_confidence的ArrayRef中。然后,使用这个Value创建一个新的Node,名为newNode,类型为kBoundingBoxSize。接着,创建一个名为anchorGrid的Value,调用createAnchorGridCoeff函数,将其与newNode连接起来。然后,更新newNode的输出大小和名称,并将其插入mul1之前。最后,将mul2和pow替换为newNode,并将mul1销毁。函数最后返回true。
这段代码的具体作用需要结合整个程序的上下文才能准确理解。
阅读全文
相关推荐
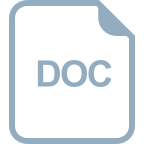
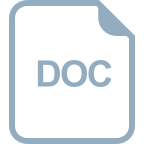
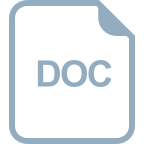
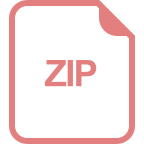
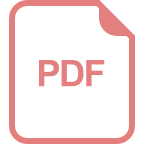
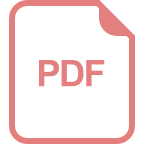




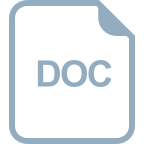
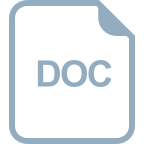
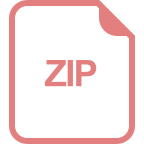
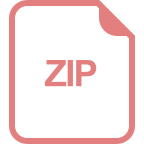
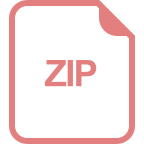
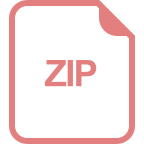
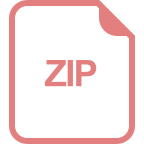