用c语言构造一个链表用来存放集合数据
时间: 2023-05-26 21:05:23 浏览: 85
链表的数据结构通常包括一个指向下一个节点的指针和一个存储数据的数据项。其中,头节点是链表的开始,尾节点没有下一个节点的指针。
下面是一个简单的 C 语言程序示例,用于构造一个链表并添加/删除元素:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表的节点结构
struct Node {
int data; // 存储的数据项
struct Node* next; // 指向下一个节点的指针
};
// 构造一个新的节点
struct Node* newNode(int data) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
return node;
}
// 在链表的尾部添加一个节点
void appendNode(struct Node* head, int data) {
struct Node* node = newNode(data);
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
// 在链表中查找某个数据项
int findNode(struct Node* head, int data) {
struct Node* current = head;
while (current != NULL) {
if (current->data == data) {
return 1;
}
current = current->next;
}
return 0;
}
// 从链表中删除某个数据项
void deleteNode(struct Node* head, int data) {
struct Node* prev = head;
struct Node* current = head->next;
while (current != NULL) {
if (current->data == data) {
prev->next = current->next;
free(current);
return;
}
prev = current;
current = current->next;
}
}
// 输出链表中的所有元素
void printList(struct Node* head) {
struct Node* current = head->next;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
struct Node* head = newNode(0);
// 向链表尾部添加元素
appendNode(head, 1);
appendNode(head, 2);
appendNode(head, 3);
// 输出链表中的元素
printList(head);
// 查找元素
int search1 = findNode(head, 2);
printf("Is 2 in the list? %d\n", search1);
int search2 = findNode(head, 5);
printf("Is 5 in the list? %d\n", search2);
// 从链表中删除元素
deleteNode(head, 2);
// 再次输出链表中的元素
printList(head);
return 0;
}
```
在这个示例程序中,`head` 是链表的头节点,它存储的数据项为 `0`,代表链表的开始。`newNode` 函数用于构造一个新的节点,`appendNode` 函数用于将一个新的节点添加到链表的尾部,`findNode` 函数用于查找链表中是否存在某个数据项,`deleteNode` 函数用于从链表中删除某个数据项,`printList` 函数用于输出链表中的所有元素。
在 `main` 函数中,我们首先创建了一个新的链表,并向链表中添加了三个元素。然后,我们调用 `findNode` 函数来查找链表中是否存在数据项 `2` 和 `5`。接着,我们调用 `deleteNode` 函数来从链表中删除数据项 `2`,然后再次输出链表中的所有元素。
阅读全文
相关推荐
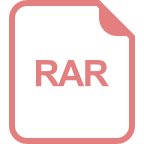
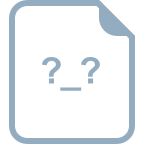
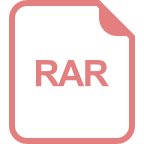
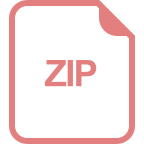
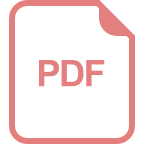
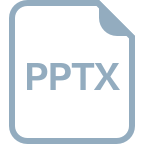
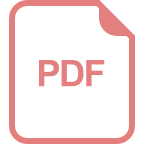
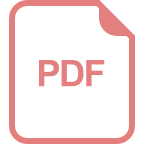
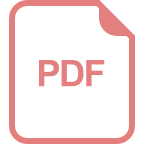
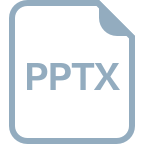
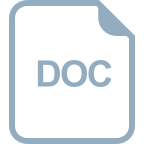
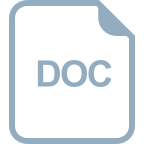
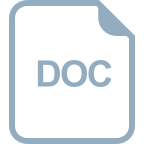
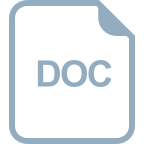
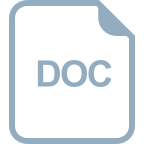
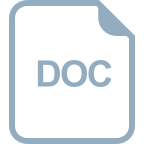
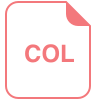