多线程处理可以改进Windows窗体应用的性能,但对Windows窗体控件的访问本质上不是线程安全的。如果要在应用中实现多线程处理,应该以线程安全的方式调用跨线程控件。请举例说明如何实现
时间: 2024-02-01 11:14:55 浏览: 30
在Windows窗体应用程序中,如果多个线程需要同时访问同一个控件(如Label、TextBox等),就需要使用线程安全的方法来访问控件,以避免出现访问冲突和其他线程安全问题。
以下是一个示例,展示如何以线程安全的方式访问跨线程控件:
```csharp
public partial class Form1 : Form
{
private delegate void UpdateTextDelegate(string text);
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// 创建一个新线程
Thread thread = new Thread(new ThreadStart(ThreadMethod));
thread.Start();
}
private void ThreadMethod()
{
// 线程执行的代码
// ...
// 调用UpdateText方法更新跨线程控件
string text = "Hello from thread " + Thread.CurrentThread.ManagedThreadId;
UpdateText(text);
}
private void UpdateText(string text)
{
// 如果当前线程不是UI线程,则通过Invoke方法调用UpdateTextDelegate委托
if (this.InvokeRequired)
{
UpdateTextDelegate updateText = new UpdateTextDelegate(UpdateText);
this.Invoke(updateText, new object[] { text });
}
else
{
// 否则直接更新控件
label1.Text = text;
}
}
}
```
在上面的示例中,我们创建了一个新线程,并在该线程中调用了ThreadMethod方法。在ThreadMethod方法中,我们调用了UpdateText方法来更新跨线程控件。在UpdateText方法中,我们首先检查当前线程是否是UI线程,如果不是,则通过Invoke方法调用UpdateTextDelegate委托来在UI线程中更新控件;如果是,则直接更新控件。
通过这种方式,我们可以实现多线程处理,并且以线程安全的方式访问跨线程控件。
相关推荐
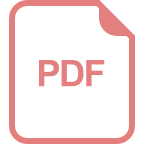
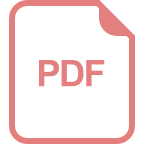














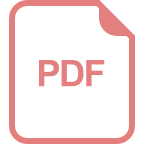