vuex mapactions 使用教程
时间: 2023-05-31 07:20:12 浏览: 123
### 回答1:
Vuex mapActions 使用教程:
1. 在组件中引入 Vuex:
```
import Vuex from 'vuex'
Vue.use(Vuex)
```
2. 创建存储实例:
```
const store = new Vuex.Store({
state: { ... },
mutations: { ... },
actions: { ... }
})
```
3. 使用 mapActions 辅助函数:
```
import { mapActions } from 'vuex'
```
4. 在组件中使用 mapActions:
```
export default {
// ...
methods: {
...mapActions(['action1', 'action2']),
// 或者
...mapActions({
a1: 'action1',
a2: 'action2'
})
}
}
```
5. 调用存储中的动作:
```
this.action1()
this.a2()
```
6. 如果需要传递参数,可以在调用动作时传递:
```
this.action1(payload)
this.a2({
param1,
param2
})
```
### 回答2:
Vuex 是 Vue.js 官方的状态管理库,它可以让我们更好地管理组件状态的包括响应式数据、计算属性、触发方法等。Vuex 提供了一批辅助函数来简化对 store 的访问,其中一个常用的辅助函数是 mapActions,它可以将 store 中的 action 映射到组件的方法中,方便我们调用。
使用 mapActions,需要首先在 Vue 组件中引入它:
```javascript
import { mapActions } from 'vuex'
```
然后,在 Vue 组件的 computed 属性中定义映射方法:
```javascript
computed: {
...mapActions([
'increment',
'decrement',
])
}
```
这个例子中,我们使用了 spread (展开)操作符来将 mapActions 返回的对象合并到 computed 对象中。注意,我们传递的数组里面的字符串是 action 的名称,即 store 中定义的方法。
最后,在我们的组件方法中,可以使用映射方法调用 action:
```javascript
methods: {
...mapActions([
'increment',
'decrement',
]),
add() {
this.increment();
},
reduce() {
this.decrement();
}
}
```
这个例子中,我们定义了 add 和 reduce 方法,并在方法中通过 this.increment() 和 this.decrement() 调用了映射的 action。
总的来说,Vuex 的 mapActions 函数让我们不需要在组件中手动调用 $store.dispatch 方法,而是把 action 作为一个映射函数传递进去,使组件可以直接调用 store 中的方法,显著简化了组件的代码量,提高了开发效率。
### 回答3:
Vuex是Vue.js应用程序开发的状态管理模式,提供集中式存储管理,方便管理Vue应用的所有组件。Vuex中提供了一些方法,如mapActions(),可以将actions映射到组件的method中,以便简化组件中的代码。下面是Vuex mapActions的使用教程。
使用方法:
1.导入Vuex库和需要操作的actions。
```
import { mapActions } from "vuex";
import { NAME_OF_ACTIONS } from "../vuex/actions.js";
```
2.定义好组件的methods。
```
methods: {
method1() {},
method2() {},
}
```
3.使用mapActions方法映射actions到组件的methods中。
```
methods: {
...mapActions({
action1: NAME_OF_ACTIONS.ACTION1,
action2: NAME_OF_ACTIONS.ACTION2,
}),
method1() {},
method2() {},
}
```
4.在组件中使用定义好的方法。
```
<button @click="action1()">执行action1</button>
<button @click="action2()">执行action2</button>
```
以上就是Vuex mapActions的使用教程,这个方法可以让我们更方便地获取Vuex中的actions,提高代码的可读性和可维护性。
阅读全文
相关推荐
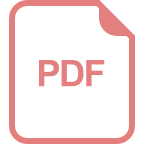
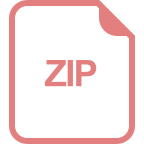
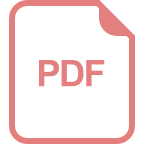









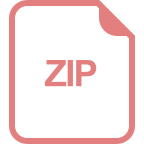
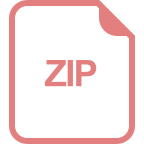
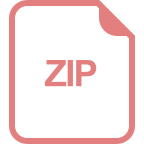
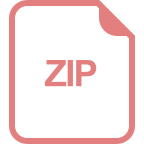
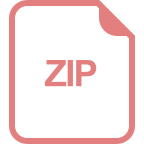
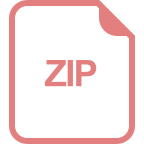
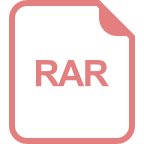