灰狼算法优化bp网络,写出相关代码
时间: 2023-07-07 12:28:06 浏览: 102
下面是一个使用灰狼算法优化BP神经网络的示例代码:
```python
import numpy as np
# 定义 BP 神经网络类
class BPNetwork:
def __init__(self, input_size, hidden_size, output_size):
# 初始化权重和偏置
self.weights1 = np.random.randn(hidden_size, input_size)
self.bias1 = np.random.randn(hidden_size, 1)
self.weights2 = np.random.randn(output_size, hidden_size)
self.bias2 = np.random.randn(output_size, 1)
# 定义 sigmoid 激活函数
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
# 定义前向传播函数
def forward(self, x):
h = self.sigmoid(np.dot(self.weights1, x) + self.bias1)
y = np.dot(self.weights2, h) + self.bias2
return y, h
# 定义误差平方和函数
def loss(self, y_pred, y_true):
return np.sum((y_pred - y_true) ** 2)
# 定义灰狼算法优化函数
def optimize(self, X, Y, iterations=100, population_size=10, alpha=0.5, beta=0.2, delta=0.2):
# 初始化灰狼群体
wolves = np.random.randn(population_size, self.weights1.size + self.weights2.size)
# 开始迭代
for i in range(iterations):
# 计算适应度函数
fitness = np.zeros(population_size)
for j in range(population_size):
self.weights1 = np.reshape(wolves[j, :self.weights1.size], self.weights1.shape)
self.bias1 = np.reshape(wolves[j, self.weights1.size:self.weights1.size+self.bias1.size], self.bias1.shape)
self.weights2 = np.reshape(wolves[j, self.weights1.size+self.bias1.size:self.weights1.size+self.bias1.size+self.weights2.size], self.weights2.shape)
self.bias2 = np.reshape(wolves[j, self.weights1.size+self.bias1.size+self.weights2.size:], self.bias2.shape)
y_pred, _ = self.forward(X.T)
fitness[j] = 1 / (1 + self.loss(y_pred, Y.T))
# 更新灰狼位置
alpha_wolf = np.argmax(fitness)
for j in range(population_size):
r1 = np.random.rand(self.weights1.size + self.bias1.size + self.weights2.size + self.bias2.size)
r2 = np.random.rand(self.weights1.size + self.bias1.size + self.weights2.size + self.bias2.size)
A = 2 * r1 * alpha_wolf - r1
C = 2 * r2 - 1
D_alpha = np.abs(beta * wolves[alpha_wolf, :] - wolves[j, :])
X1 = wolves[alpha_wolf, :] - A * D_alpha
D_beta = np.abs(beta * wolves[np.argmax(fitness), :] - wolves[j, :])
X2 = wolves[np.argmax(fitness), :] - A * D_beta
D_delta = np.abs(beta * wolves[np.random.randint(population_size), :] - wolves[j, :])
X3 = wolves[np.random.randint(population_size), :] - A * D_delta
wolves[j, :] = (X1 + X2 + X3) / 3
# 更新最优解
self.weights1 = np.reshape(wolves[alpha_wolf, :self.weights1.size], self.weights1.shape)
self.bias1 = np.reshape(wolves[alpha_wolf, self.weights1.size:self.weights1.size+self.bias1.size], self.bias1.shape)
self.weights2 = np.reshape(wolves[alpha_wolf, self.weights1.size+self.bias1.size:self.weights1.size+self.bias1.size+self.weights2.size], self.weights2.shape)
self.bias2 = np.reshape(wolves[alpha_wolf, self.weights1.size+self.bias1.size+self.weights2.size:], self.bias2.shape)
# 输出当前迭代次数和误差
y_pred, _ = self.forward(X.T)
print("Iteration: %d, Loss: %f" % (i, self.loss(y_pred, Y.T)))
# 测试代码
if __name__ == '__main__':
# 生成训练数据
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
Y = np.array([[0], [1], [1], [0]])
# 创建 BP 神经网络实例
network = BPNetwork(2, 4, 1)
# 使用灰狼算法优化网络
network.optimize(X, Y, iterations=100)
# 输出最终预测结果
y_pred, _ = network.forward(X.T)
print("Predictions: ", y_pred)
```
需要注意的是,这只是一个简单的示例,实际应用时需要针对具体问题进行调整和优化。
阅读全文
相关推荐
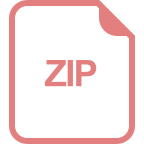
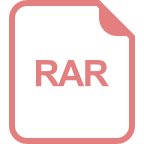
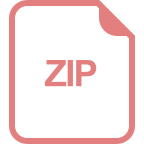
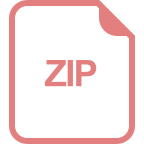
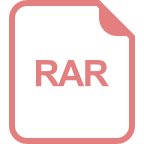
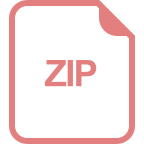
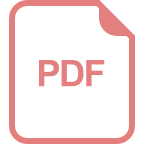
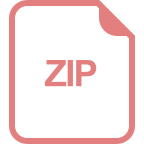
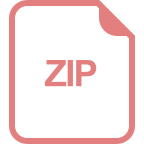
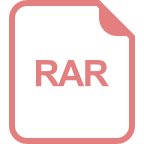
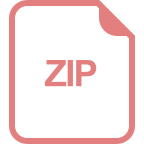
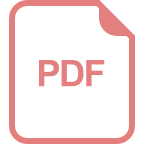
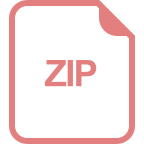
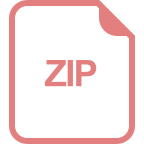
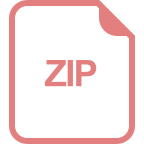
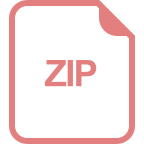
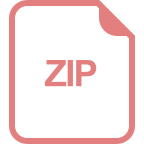
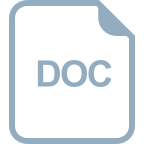