写出c++代码:Design a Student Grade Management Program with C++ STL (Phase 1) Data Structures: class CStudent, includes the following data members: nID (integer) (学号) strName (string) (学生姓名) mapScores (Store the course numbers (integer) and scores (double) of all courses using a map container) 注:mapScores(用map容器存储修读过的所有课程的课程号(整型)和成绩(double)) Data storage: store all students' data in some kind of appropriate STL container. 注:用某种适当的STL容器存储所有学生的数据 Add three student data (each student takes several courses) to the container, and output the information of all students in the container. Data maintenance: Implement the functions of adding, deleting, modifying, and querying student data. 注:实现对学生数据的数据维护,包括增、删、改、查功能(增、删、改、查学生信息,以及各科成绩)
时间: 2023-06-02 17:05:01 浏览: 102
抱歉,我可以回答您的问题。以下是一个简单的C++代码,用于创建一个包含学生姓名、学号和各科成绩的成绩管理程序:
#include <iostream>
#include <string>
#include <map>
#include <vector>
class CStudent {
public:
CStudent() {}
CStudent(int id, std::string name) : nID(id), strName(name) {}
int getID() const { return nID; }
std::string getName() const { return strName; }
void addScore(int courseID, double score) { mapScores[courseID] = score; }
double getScore(int courseID) const {
auto it = mapScores.find(courseID);
if (it != mapScores.end()) {
return it->second;
} else {
return -1.0;
}
}
void print() const {
std::cout << "ID: " << nID << ", Name: " << strName << "\n";
std::cout << "Scores: \n";
for (auto it = mapScores.begin(); it != mapScores.end(); ++it) {
std::cout << " Course " << it->first << ": " << it->second << "\n";
}
}
private:
int nID;
std::string strName;
std::map<int, double> mapScores;
};
class CGradeManager {
public:
void addStudent(const CStudent& student) {
students.push_back(student);
}
void removeStudent(int id) {
for (auto it = students.begin(); it != students.end(); ++it) {
if (it->getID() == id) {
students.erase(it);
return;
}
}
}
void updateStudent(const CStudent& student) {
for (auto it = students.begin(); it != students.end(); ++it) {
if (it->getID() == student.getID()) {
*it = student;
return;
}
}
}
CStudent getStudent(int id) const {
for (auto it = students.begin(); it != students.end(); ++it) {
if (it->getID() == id) {
return *it;
}
}
return CStudent();
}
void printAll() const {
for (auto it = students.begin(); it != students.end(); ++it) {
it->print();
std::cout << "\n";
}
}
private:
std::vector<CStudent> students;
};
int main() {
CGradeManager manager;
CStudent student1(123, "Tom");
student1.addScore(1, 80);
student1.addScore(2, 90);
student1.addScore(3, 85);
CStudent student2(456, "Jerry");
student2.addScore(1, 70);
student2.addScore(2, 95);
student2.addScore(4, 90);
CStudent student3(789, "Alice");
student3.addScore(1, 95);
student3.addScore(3, 90);
manager.addStudent(student1);
manager.addStudent(student2);
manager.addStudent(student3);
std::cout << "All Students:\n";
manager.printAll();
std::cout << "\n";
CStudent student4(123, "Tim");
student4.addScore(1, 85);
manager.updateStudent(student4);
std::cout << "After Update:\n";
manager.printAll();
std::cout << "\n";
manager.removeStudent(789);
std::cout << "After Remove:\n";
manager.printAll();
std::cout << "\n";
return 0;
}
阅读全文
相关推荐
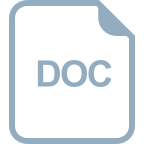
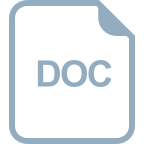
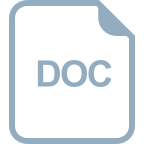
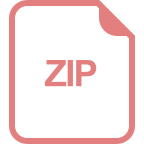
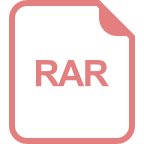
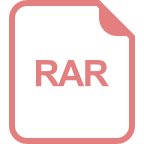
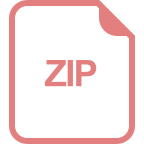
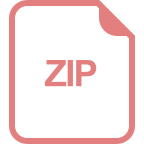
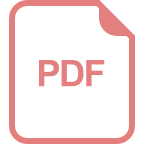
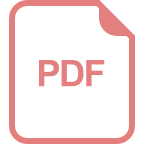
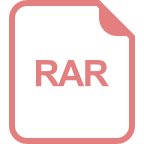
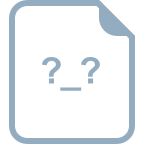
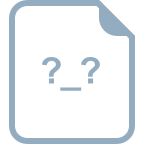
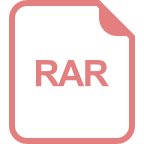
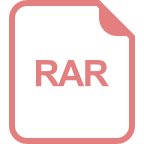
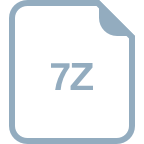
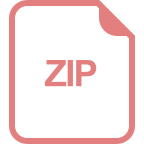
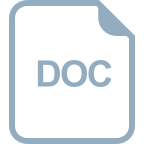
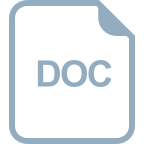