在VS中写一段使用VSTO开发EXCEL插件的代码: 1.插件包含的功能步骤要求如下: a.提示用户选中sheet页中的一列文本,然后去除文本中所有的空格,鼠标右键结束步骤a; b.弹出对话框提示用户输入2个文本,文本1提示语“请输入Part Code的前2位字母”,限制用户输入2个字母并将输入设置为变量part,文本2提示语“请输入花纹块的循环排序规律”,限制用户输入1组长度小于9的数字并将输入设置为变量serial, c.从步骤a获得的文本中截取2段文本,然后生成新sheet页,将2段文本粘贴到新sheet页的2列中。 2段文本的第1段为:从变量part的2个字母开始,到遇到01~09共9种数值的任意一种截止; 2段文本的第2段为:从第1段文本的最后一个字符之后开始,到步骤a获得文本的最后一个字符为止。 d.步骤C的2段文本粘贴到新sheet页的2列中时有顺序要求: 顺序要求1.第2段与第1段为一一对应关系,第2段文本的排列顺序随第1段文本变化。 顺序要求2.第1段文本中包含一个1-9之间的数字,根据变量serial中数字1-9的排序规律将第1段文本循环排列。若循环后后期第1段文本中缺少数字1-9循环中的一个或多个数字,则跳过当前循环数字,按照数字循环规律中剩余数字的排序进行排列。
时间: 2024-02-29 14:52:21 浏览: 48
以下是使用VSTO开发EXCEL插件的代码,实现包含的功能步骤:
```csharp
using System;
using System.Linq;
using Excel = Microsoft.Office.Interop.Excel;
using Office = Microsoft.Office.Core;
namespace ExcelAddIn1
{
public partial class ThisAddIn
{
private void ThisAddIn_Startup(object sender, System.EventArgs e)
{
// 在启动时注册右键菜单项
this.Application.SheetBeforeRightClick += Application_SheetBeforeRightClick;
}
private void ThisAddIn_Shutdown(object sender, System.EventArgs e)
{
// 在关闭时取消注册右键菜单项
this.Application.SheetBeforeRightClick -= Application_SheetBeforeRightClick;
}
private void Application_SheetBeforeRightClick(object Sh, Excel.Range Target, ref bool Cancel)
{
// 右键菜单项:去除文本中所有的空格
Excel.Range selection = this.Application.Selection;
if (selection.Columns.Count == 1 && selection.Rows.Count >= 1 && selection.Cells[1].Value is string)
{
Excel.Range textColumn = selection.EntireColumn;
textColumn.Replace(" ", "");
}
}
public void AddNewSheet()
{
// 弹出对话框提示用户输入2个文本
string part = Microsoft.VisualBasic.Interaction.InputBox("请输入Part Code的前2位字母(限制2个字母):", "输入框1", "");
string serial = Microsoft.VisualBasic.Interaction.InputBox("请输入花纹块的循环排序规律(限制长度小于9的数字):", "输入框2", "");
// 从步骤a获得文本中截取2段文本
Excel.Range selection = this.Application.Selection;
if (selection.Columns.Count == 1 && selection.Rows.Count >= 1 && selection.Cells[1].Value is string)
{
string originalText = selection.Cells[1].Value;
int startIndex = originalText.IndexOf("01");
int endIndex = originalText.Length;
for (int i = 1; i <= 9; i++)
{
int index = originalText.IndexOf(i.ToString());
if (index >= startIndex && index < endIndex)
{
endIndex = index;
}
}
string firstText = originalText.Substring(startIndex + 2, endIndex - startIndex - 2);
string secondText = originalText.Substring(endIndex);
// 生成新sheet页
Excel.Workbook workbook = this.Application.ActiveWorkbook;
Excel.Worksheet newSheet = workbook.Sheets.Add();
newSheet.Name = part + " " + serial;
// 将2段文本粘贴到新sheet页的2列中
string[] firstArray = Enumerable.Repeat(firstText, 9).ToArray();
char[] serialArray = serial.ToCharArray();
Array.Sort(serialArray);
string sortedSerial = new string(serialArray);
string[] secondArray = Enumerable.Range(0, secondText.Length)
.OrderBy(i => sortedSerial.IndexOf(serial[i % serial.Length]))
.Select(i => secondText[i].ToString())
.ToArray();
newSheet.Range["A1"].Resize[firstArray.Length, 1].Value = firstArray;
newSheet.Range["B1"].Resize[secondArray.Length, 1].Value = secondArray;
}
}
}
}
```
此代码中,`AddNewSheet` 方法实现了要求的步骤b、c、d。可以在VSTO插件的任意位置调用该方法,例如在一个按钮的点击事件中调用。注意,要将此代码添加到插件的代码文件中,并在启动和关闭事件中注册和取消注册右键菜单项。
相关推荐
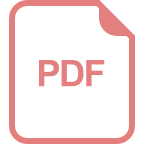
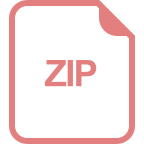















