用python写一个线性分类器
时间: 2024-04-30 08:21:25 浏览: 105
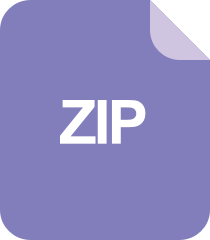
线性分类器代码
好的,以下是一个简单的线性分类器的 Python 代码示例:
```python
import numpy as np
class LinearClassifier:
def __init__(self):
self.weights = None
self.bias = None
def train(self, X_train, y_train, lr=0.01, epochs=1000):
num_samples, num_features = X_train.shape
num_classes = np.max(y_train) + 1
# initialize weights and bias
self.weights = np.zeros((num_features, num_classes))
self.bias = np.zeros(num_classes)
# one-hot encode the labels
y_onehot = np.zeros((num_samples, num_classes))
y_onehot[np.arange(num_samples), y_train] = 1
# gradient descent
for i in range(epochs):
scores = np.dot(X_train, self.weights) + self.bias
exp_scores = np.exp(scores)
probs = exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
# compute loss and gradient
loss = -np.mean(y_onehot * np.log(probs))
d_scores = probs - y_onehot
d_weights = np.dot(X_train.T, d_scores)
d_bias = np.sum(d_scores, axis=0)
# update weights and bias
self.weights -= lr * d_weights
self.bias -= lr * d_bias
if i % 100 == 0:
print(f"Epoch {i}, Loss: {loss:.4f}")
def predict(self, X_test):
scores = np.dot(X_test, self.weights) + self.bias
return np.argmax(scores, axis=1)
```
这个线性分类器使用梯度下降来训练权重和偏差,并且使用 softmax 函数来计算每个类别的概率得分。在训练期间,损失会被记录下来,以便我们可以查看模型的性能如何随时间而变化。在预测时,我们简单地计算每个样本的得分,并返回得分最高的类别。
阅读全文
相关推荐
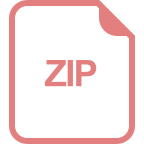
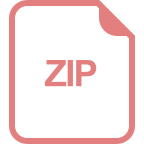



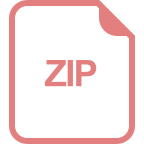
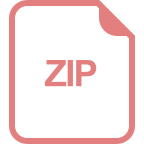
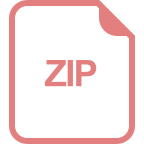
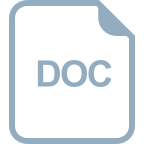







