vue3使用axios前后端连接
时间: 2023-09-17 13:12:18 浏览: 50
1. 安装axios
使用npm安装axios
```
npm install axios
```
2. 创建axios实例
在Vue3中,我们可以通过createApp方法来创建一个Vue实例,同样的,在使用axios时,我们可以通过create方法来创建一个axios实例。在创建axios实例时,我们可以设置一些默认的配置,例如baseURL、headers等。
```javascript
import axios from 'axios'
const instance = axios.create({
baseURL: 'http://localhost:3000',
timeout: 10000,
headers: {
'Content-Type': 'application/json'
}
})
```
3. 发送请求
我们可以通过axios实例的get、post等方法来发送请求。例如:
```javascript
// get请求
instance.get('/api/users').then(res => {
console.log(res.data)
}).catch(err => {
console.log(err)
})
// post请求
const data = {
username: 'test',
password: '123456'
}
instance.post('/api/login', data).then(res => {
console.log(res.data)
}).catch(err => {
console.log(err)
})
```
4. 使用async/await异步处理请求
我们可以使用async/await来异步处理请求,并且可以使用try/catch语句来捕获错误。例如:
```javascript
async function getUsers() {
try {
const res = await instance.get('/api/users')
console.log(res.data)
} catch (err) {
console.log(err)
}
}
async function login() {
try {
const data = {
username: 'test',
password: '123456'
}
const res = await instance.post('/api/login', data)
console.log(res.data)
} catch (err) {
console.log(err)
}
}
```
相关推荐
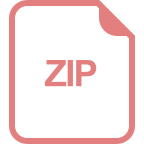
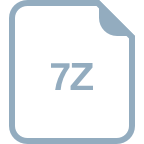
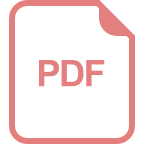
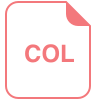
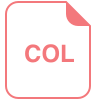
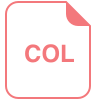
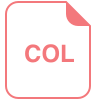










