写一个Linux c语言打印指定目录下文件名的函数
时间: 2024-03-20 14:42:50 浏览: 14
可以使用 `opendir()` 和 `readdir()` 函数来实现该功能。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
void list_files(const char *path) {
DIR *dir;
struct dirent *entry;
dir = opendir(path);
if (dir == NULL) {
perror("opendir");
exit(EXIT_FAILURE);
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) { // 如果是普通文件
printf("%s\n", entry->d_name);
}
}
closedir(dir);
}
```
在该函数中,首先通过 `opendir()` 函数打开指定目录,如果打开失败,则输出错误信息并退出程序。然后通过 `readdir()` 函数循环读取目录下的文件项,对于每个文件项,如果是一个普通文件,则输出文件名。最后通过 `closedir()` 函数关闭目录。需要注意的是,这里只输出了普通文件的文件名,如果需要输出其他类型的文件名,需要修改 `if` 语句中的条件判断。
相关问题
写一个Linux c语言函数,对于指定路径是文件时,打印文件名,是目录时,打印目录下所有文件文件名
以下是一个简单的Linux C语言函数,可以实现对指定路径的判断,如果是文件,则打印文件名;如果是目录,则打印目录下所有文件名:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <sys/stat.h>
void print_files(char *path) {
DIR *dir;
struct dirent *entry;
struct stat file_stat;
dir = opendir(path);
if (dir == NULL) {
printf("Unable to open directory: %s\n", path);
return;
}
while ((entry = readdir(dir)) != NULL) {
char fullpath[512];
sprintf(fullpath, "%s/%s", path, entry->d_name);
if (lstat(fullpath, &file_stat) == -1) {
printf("Unable to stat file: %s\n", fullpath);
continue;
}
if (S_ISREG(file_stat.st_mode)) {
printf("File: %s\n", entry->d_name);
} else if (S_ISDIR(file_stat.st_mode)) {
if (strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) {
printf("Directory: %s\n", entry->d_name);
print_files(fullpath);
}
}
}
closedir(dir);
}
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s [path]\n", argv[0]);
return 0;
}
print_files(argv[1]);
return 0;
}
```
这个函数接受一个路径作为参数,使用`opendir`函数打开目录,然后使用`readdir`函数遍历目录下的所有文件和目录。对于每个文件或目录,使用`lstat`函数获取文件属性,然后根据属性判断是文件还是目录。如果是文件,则直接打印文件名;如果是目录,则递归调用`print_files`函数,打印目录下的所有文件名。
linux c语言遍历目录并文件名
您可以使用以下代码来遍历目录并输出文件名:
```c
#include <stdio.h>
#include <dirent.h>
void traverse_dir(char *dir_path) {
DIR *dir;
struct dirent *entry;
if ((dir = opendir(dir_path)) == NULL) {
perror("opendir");
return;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_DIR) {
// Ignore "." and ".." directories
if (!strcmp(entry->d_name, ".") || !strcmp(entry->d_name, "..")) {
continue;
}
char subdir_path[512];
snprintf(subdir_path, sizeof(subdir_path), "%s/%s", dir_path, entry->d_name);
traverse_dir(subdir_path);
} else {
printf("%s/%s\n", dir_path, entry->d_name);
}
}
closedir(dir);
}
int main() {
traverse_dir(".");
return 0;
}
```
这个程序首先打开目录,然后使用 `readdir` 函数逐个读取目录中的条目。如果条目是一个目录,则递归调用 `traverse_dir` 函数来遍历该目录。如果条目是一个文件,则输出该文件的路径。注意,该程序假设当前目录是要遍历的目录,您可以修改 `traverse_dir` 函数的参数来指定要遍历的目录。
相关推荐
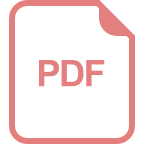
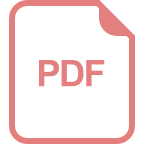
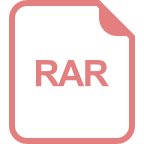












