用verliog写一个五级流水线cpu
时间: 2023-12-20 14:04:51 浏览: 189
设计一个完整的五级流水线CPU比较复杂,需要考虑到许多细节,这里提供一个基本的框架,仅供参考:
```verilog
// 定义指令宽度
parameter INSTRUCTION_WIDTH = 32;
// 定义寄存器宽度
parameter REGISTER_WIDTH = 32;
// 定义指令类型
parameter [2:0] INSTRUCTION_TYPE = 3'b000; //例如,R型指令为000
module pipeline_cpu(
input clk,
input reset,
input [INSTRUCTION_WIDTH-1:0] instruction_in,
output [REGISTER_WIDTH-1:0] data_out
);
// 定义寄存器堆
reg [REGISTER_WIDTH-1:0] registers [31:0];
// 定义流水线寄存器
reg [INSTRUCTION_WIDTH-1:0] if_id_instruction;
reg [INSTRUCTION_WIDTH-1:0] id_ex_instruction;
reg [INSTRUCTION_WIDTH-1:0] ex_mem_instruction;
reg [INSTRUCTION_WIDTH-1:0] mem_wb_instruction;
// 定义流水线寄存器控制信号
reg id_ex_reg_write;
reg [4:0] id_ex_alu_src;
reg [4:0] id_ex_alu_op;
reg [4:0] id_ex_mem_write;
reg [4:0] id_ex_mem_read;
reg [4:0] ex_mem_mem_write;
reg [4:0] ex_mem_mem_read;
reg [4:0] mem_wb_reg_write;
reg [4:0] mem_wb_mem_to_reg;
reg [4:0] mem_wb_alu_op;
// 定义流水线寄存器读端口
wire [REGISTER_WIDTH-1:0] rs_read_data;
wire [REGISTER_WIDTH-1:0] rt_read_data;
// 定义ALU
wire [REGISTER_WIDTH-1:0] alu_out;
// 定义数据存储器
reg [31:0] data_memory [1023:0];
// 定义指令解码模块
decode_module decode(
.instruction(if_id_instruction),
.rs_read_data(rs_read_data),
.rt_read_data(rt_read_data),
.id_ex_reg_write(id_ex_reg_write),
.id_ex_alu_src(id_ex_alu_src),
.id_ex_alu_op(id_ex_alu_op),
.id_ex_mem_write(id_ex_mem_write),
.id_ex_mem_read(id_ex_mem_read)
);
// 定义ALU模块
alu_module alu(
.rs_data(rs_read_data),
.rt_data(rt_read_data),
.alu_op(id_ex_alu_op),
.alu_out(alu_out)
);
// 定义数据存储器模块
data_memory_module data_memory(
.address(ex_mem_instruction[19:2]),
.write_data(alu_out),
.mem_write(ex_mem_mem_write),
.mem_read(ex_mem_mem_read),
.read_data(data_out)
);
// 定义寄存器堆读写模块
register_file_module register_file(
.rs(ex_mem_instruction[25:21]),
.rt(ex_mem_instruction[20:16]),
.write_data(data_out),
.write_enable(ex_mem_reg_write),
.read_data_1(rs_read_data),
.read_data_2(rt_read_data)
);
// 定义流水线寄存器模块
always @(posedge clk) begin
if(reset) begin
if_id_instruction <= 0;
id_ex_instruction <= 0;
ex_mem_instruction <= 0;
mem_wb_instruction <= 0;
end else begin
if_id_instruction <= instruction_in;
id_ex_instruction <= if_id_instruction;
ex_mem_instruction <= id_ex_instruction;
mem_wb_instruction <= ex_mem_instruction;
end
end
// 定义流水线寄存器控制信号模块
always @(*) begin
case(id_ex_instruction[31:26])
6'b000000: begin // R型指令
id_ex_reg_write = 1;
id_ex_alu_src = 2'b00;
id_ex_alu_op = id_ex_instruction[5:0];
id_ex_mem_write = 0;
id_ex_mem_read = 0;
ex_mem_mem_write = 0;
ex_mem_mem_read = 0;
mem_wb_reg_write = 1;
mem_wb_mem_to_reg = 0;
mem_wb_alu_op = 0;
end
// 其他指令类型的控制信号
default: begin
id_ex_reg_write = 0;
id_ex_alu_src = 2'b00;
id_ex_alu_op = 0;
id_ex_mem_write = 0;
id_ex_mem_read = 0;
ex_mem_mem_write = 0;
ex_mem_mem_read = 0;
mem_wb_reg_write = 0;
mem_wb_mem_to_reg = 0;
mem_wb_alu_op = 0;
end
endcase
end
// 最终输出结果
assign data_out = mem_wb_instruction[15:0] == 0 ? 0 : registers[mem_wb_instruction[20:16]];
endmodule
// 定义指令解码模块
module decode_module(
input [INSTRUCTION_WIDTH-1:0] instruction,
input [REGISTER_WIDTH-1:0] rs_read_data,
input [REGISTER_WIDTH-1:0] rt_read_data,
output reg reg_write,
output reg [4:0] alu_src,
output reg [4:0] alu_op,
output reg [4:0] mem_write,
output reg [4:0] mem_read
);
// 从指令中解码出寄存器编号和控制信号
endmodule
// 定义ALU模块
module alu_module(
input [REGISTER_WIDTH-1:0] rs_data,
input [REGISTER_WIDTH-1:0] rt_data,
input [3:0] alu_op,
output reg [REGISTER_WIDTH-1:0] alu_out
);
// 根据控制信号执行相应的操作
endmodule
// 定义数据存储器模块
module data_memory_module(
input [9:0] address,
input [REGISTER_WIDTH-1:0] write_data,
input mem_write,
input mem_read,
output reg [REGISTER_WIDTH-1:0] read_data
);
// 根据控制信号执行相应的操作
endmodule
// 定义寄存器堆读写模块
module register_file_module(
input [4:0] rs,
input [4:0] rt,
input [REGISTER_WIDTH-1:0] write_data,
input write_enable,
output reg [REGISTER_WIDTH-1:0] read_data_1,
output reg [REGISTER_WIDTH-1:0] read_data_2
);
// 根据控制信号执行相应的操作
endmodule
```
以上仅是一个大致的框架,具体实现还需要更多的细节处理。
阅读全文
相关推荐
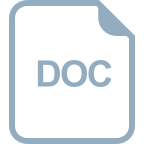
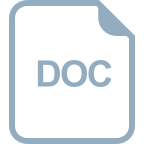
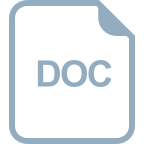
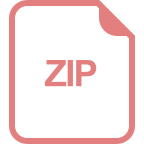
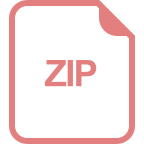



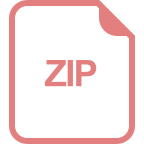
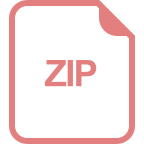
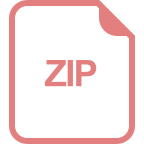
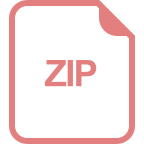
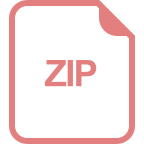
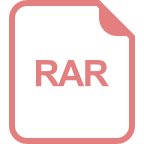
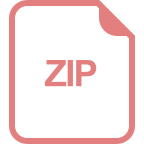
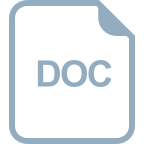
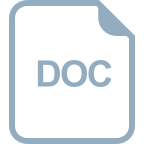
