python如何给串口加锁
时间: 2023-07-19 10:33:01 浏览: 92
在Python中,可以使用`fcntl`和`termios`模块来给串口加锁。
以下是一个简单的示例代码:
```
import fcntl
import termios
import serial
# 打开串口
ser = serial.Serial('/dev/ttyUSB0', 9600)
# 获取串口文件描述符
fd = ser.fileno()
# 设置串口属性
attrs = termios.tcgetattr(fd)
attrs[2] |= termios.HUPCL
termios.tcsetattr(fd, termios.TCSANOW, attrs)
# 加锁
fcntl.flock(fd, fcntl.LOCK_EX)
# 发送数据
ser.write(b'Hello World!')
# 解锁
fcntl.flock(fd, fcntl.LOCK_UN)
# 关闭串口
ser.close()
```
在上面的代码中,`fcntl.flock(fd, fcntl.LOCK_EX)`表示对串口进行独占式加锁,`fcntl.flock(fd, fcntl.LOCK_UN)`表示解锁。注意,加锁的作用是防止多个进程同时访问同一个串口,如果只有一个进程访问串口,则可以省略加锁的步骤。
相关问题
Python 给文件加锁
要在Python中给文件加锁,你可以使用`flock()`方法。以下是一个基本的示例代码:
```python
import fcntl
file_path = 'path/to/your/file.txt'
# 打开文件并获取文件句柄
file = open(file_path, 'a')
# 尝试给文件加锁
try:
fcntl.flock(file, fcntl.LOCK_EX | fcntl.LOCK_NB)
print("文件已加锁")
# 在这里执行对文件的操作
finally:
# 解锁文件
fcntl.flock(file, fcntl.LOCK_UN)
print("文件已解锁")
# 关闭文件
file.close()
```
在这个示例中,`fcntl.flock()`函数用于给文件加锁。`fcntl.LOCK_EX`表示独占锁,`fcntl.LOCK_NB`表示非阻塞模式。如果文件已经被其他进程锁定,且没有设置非阻塞模式,`fcntl.flock()`方法将会阻塞程序直到获取到锁为止。
在使用完文件后,一定要记得使用`fcntl.LOCK_UN`参数解除文件锁定。这样可以确保其他进程能够访问该文件。
请注意,文件锁只能对同一台机器上的不同进程起作用。如果在不同机器上运行的进程需要访问同一个文件,你可能需要考虑其他的并发控制机制。
python中给进程加锁
在 Python 中,可以使用 `multiprocessing` 模块来创建并发的进程。如果需要在多个进程之间进行数据共享或者对共享资源进行互斥访问,可以使用 `Lock` 对象来实现进程间的加锁。
下面是一个简单的示例代码,展示了如何在两个进程之间使用 `Lock` 对象进行加锁:
```python
import multiprocessing
def worker(lock, shared_data):
# 加锁
lock.acquire()
try:
# 对共享资源进行操作
shared_data.value += 1
print(f"Worker: {shared_data.value}")
finally:
# 释放锁
lock.release()
if __name__ == '__main__':
# 创建共享数据
shared_data = multiprocessing.Value('i', 0)
# 创建锁对象
lock = multiprocessing.Lock()
# 创建两个进程
p1 = multiprocessing.Process(target=worker, args=(lock, shared_data))
p2 = multiprocessing.Process(target=worker, args=(lock, shared_data))
# 启动进程
p1.start()
p2.start()
# 等待进程结束
p1.join()
p2.join()
```
在上述代码中,我们首先创建了一个共享数据 `shared_data`,它是一个整数类型的变量。然后,我们创建了一个 `Lock` 对象 `lock`,用于实现对共享数据的互斥访问。
在 `worker` 函数中,我们首先调用 `lock.acquire()` 方法来获取锁,然后对共享数据进行操作。最后,使用 `lock.release()` 方法释放锁。
注意,在使用 `Lock` 对象时,一定要保证在获取锁后,对共享资源的操作完成后再释放锁,以避免出现死锁的情况。
运行上述代码,你会发现两个进程分别对共享数据进行操作,并且通过互斥访问实现了正确的结果。
相关推荐
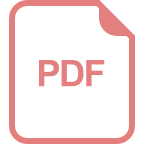












