unity开始游戏按钮代码
时间: 2023-08-24 10:38:50 浏览: 305
在 Unity 中,可以通过以下代码实现开始游戏按钮的功能:
```csharp
using UnityEngine;
using UnityEngine.SceneManagement;
public class StartGameButton : MonoBehaviour
{
public void StartGame()
{
// Load the next scene in the build order
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex + 1);
}
}
```
这个代码段创建了一个名为 `StartGameButton` 的脚本,该脚本将在开始游戏按钮上使用。 `StartGame()` 方法会在按钮被点击时调用,它通过使用 `SceneManager` 类来加载下一个场景。如果你想要加载其他场景,只需更改 `buildIndex` 的值即可。
相关问题
unity退出游戏按钮代码
在Unity中,退出游戏的代码可以使用以下C#代码实现:
```csharp
using UnityEngine;
using System.Collections;
public class ExitGame : MonoBehaviour {
public void QuitGame() {
#if UNITY_EDITOR
UnityEditor.EditorApplication.isPlaying = false;
#else
Application.Quit();
#endif
}
}
```
然后,在你的UI按钮上添加一个OnClick事件,并将QuitGame方法分配给该事件。当玩家点击该按钮时,游戏将退出。注意,如果你在Unity编辑器中运行游戏,这个代码将只是停止播放游戏,不会真正地退出游戏。
unity重新开始游戏按钮
若想在Unity中添加重新开始游戏按钮,可以通过以下步骤实现:
1. 首先,在场景中创建一个空物体来作为按钮的父物体
2. 给该空物体添加一个Button组件
3. 在场景中创建一个脚本,并将其附加到按钮的父物体上
4. 在脚本中编写重新开始游戏的代码,并将其关联到按钮的OnClick事件上
以下是一个简单的示例代码:
```csharp
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class RestartButton : MonoBehaviour
{
private Button restartButton;
// Start is called before the first frame update
void Start()
{
restartButton = GetComponent<Button>();
restartButton.onClick.AddListener(RestartGame);
}
void RestartGame()
{
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
```
这个脚本会在游戏开始时获取按钮组件,并将RestartGame方法关联到按钮的OnClick事件上。RestartGame方法会重新加载当前场景,从而实现重新开始游戏的功能。
阅读全文
相关推荐
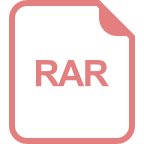
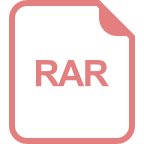
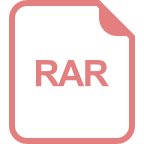













