使用Python语言手写K均值算法。
时间: 2024-02-25 19:54:20 浏览: 61
下面是使用Python手写K均值算法的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import make_blobs
class KMeans:
def __init__(self, k=3, max_iters=100):
self.k = k
self.max_iters = max_iters
self.centroids = None
def fit(self, X):
self.centroids = X[np.random.choice(X.shape[0], self.k, replace=False)]
for i in range(self.max_iters):
# Assign each data point to the closest centroid
distances = np.sqrt(((X - self.centroids[:, np.newaxis])**2).sum(axis=2))
labels = np.argmin(distances, axis=0)
# Update the centroids to be the mean of the assigned data points
for j in range(self.k):
self.centroids[j] = X[labels == j].mean(axis=0)
def predict(self, X):
distances = np.sqrt(((X - self.centroids[:, np.newaxis])**2).sum(axis=2))
return np.argmin(distances, axis=0)
# Generate sample data
X, y = make_blobs(n_samples=300, centers=3, random_state=42)
# Fit KMeans algorithm
kmeans = KMeans(k=3, max_iters=100)
kmeans.fit(X)
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=kmeans.predict(X))
plt.scatter(kmeans.centroids[:, 0], kmeans.centroids[:, 1], marker='x', s=200, linewidths=3, color='r')
plt.show()
```
这段代码使用了numpy和matplotlib库,以及scikit-learn库中的make_blobs函数生成样本数据。KMeans类包含了fit和predict两个方法,其中fit方法用于训练K均值模型,predict方法用于预测新的数据点所属的簇。在示例代码中,我们使用KMeans类对样本数据进行聚类,并将聚类结果可视化展示。
阅读全文
相关推荐
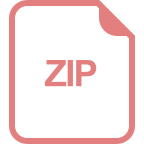
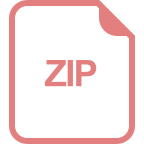
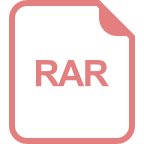
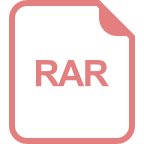
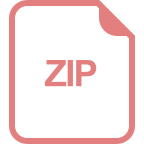
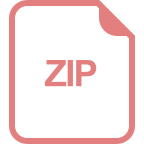
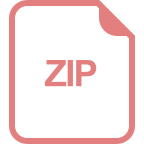
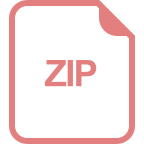