强化学习得到的tflite模型如何部署到esp32中,并利用setup和loop。其中输入为24维,输出为4维度的mean和4维度的std
时间: 2024-05-05 15:21:54 浏览: 12
将强化学习得到的tflite模型部署到ESP32中需要进行以下步骤:
1. 将tflite模型转换为TensorFlow Lite for Microcontrollers(TFLite Micro)格式。
2. 使用TFLite Micro库将模型加载到ESP32的内存中。
3. 通过ESP32的输入接口将输入数据导入到模型中,获得输出结果。
4. 处理输出结果并将其发送到其他设备。
以下是一个基本的ESP32代码示例,其中包含了将tflite模型加载到ESP32中,以及在setup和loop中使用模型进行推理的代码:
```C++
#include "tensorflow/lite/micro/all_ops_resolver.h"
#include "tensorflow/lite/micro/micro_error_reporter.h"
#include "tensorflow/lite/micro/micro_interpreter.h"
#include "tensorflow/lite/schema/schema_generated.h"
#include "tensorflow/lite/version.h"
// Include the model data
#include "model_data.h"
// Set up the input and output tensor arrays
TfLiteTensor* model_input;
TfLiteTensor* model_output_mean;
TfLiteTensor* model_output_std;
// Set up the TFLite interpreter
static tflite::MicroInterpreter* interpreter;
static tflite::MicroErrorReporter error_reporter;
static tflite::ops::micro::AllOpsResolver resolver;
// Set up the model buffer
constexpr int kModelBufferSize = 20 * 1024;
alignas(16) static uint8_t model_buffer[kModelBufferSize];
void setup() {
// Load the model into the model buffer
memcpy(model_buffer, model_data, model_data_len);
// Set up the TFLite interpreter
interpreter = new tflite::MicroInterpreter(
tflite::GetModel(model_buffer), resolver, error_reporter, /*allocated_arena=*/nullptr);
// Allocate memory for the model's input and output tensors
interpreter->AllocateTensors();
// Get pointers to the model's input and output tensors
model_input = interpreter->input(0);
model_output_mean = interpreter->output(0);
model_output_std = interpreter->output(1);
// Set up the input tensor with the correct dimensions
model_input->dims->data[0] = 1;
model_input->dims->data[1] = 24;
model_input->type = kTfLiteFloat32;
// Set up the output tensors with the correct dimensions
model_output_mean->dims->data[0] = 1;
model_output_mean->dims->data[1] = 4;
model_output_mean->type = kTfLiteFloat32;
model_output_std->dims->data[0] = 1;
model_output_std->dims->data[1] = 4;
model_output_std->type = kTfLiteFloat32;
// Set up the input data (replace with your own input data)
float input_data[24] = {...};
memcpy(model_input->data.f, input_data, 24 * sizeof(float));
}
void loop() {
// Run the inference
interpreter->Invoke();
// Get the output data
float output_mean[4];
memcpy(output_mean, model_output_mean->data.f, 4 * sizeof(float));
float output_std[4];
memcpy(output_std, model_output_std->data.f, 4 * sizeof(float));
// Process the output data (replace with your own code)
...
}
```
在这个示例中,我们将tflite模型加载到ESP32的内存中,并使用TFLite Micro库设置了模型的输入和输出张量。在setup函数中,我们设置了输入张量的维度,并将输入数据复制到该张量中。在loop函数中,我们运行了推理,并从输出张量中获取了结果。您需要将“output_mean”和“output_std”替换为您的输出张量,并修改“Process the output data”部分以便将结果发送到其他设备。
相关推荐
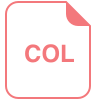













